How can you create an app to detect living objects with React? (r) (r)
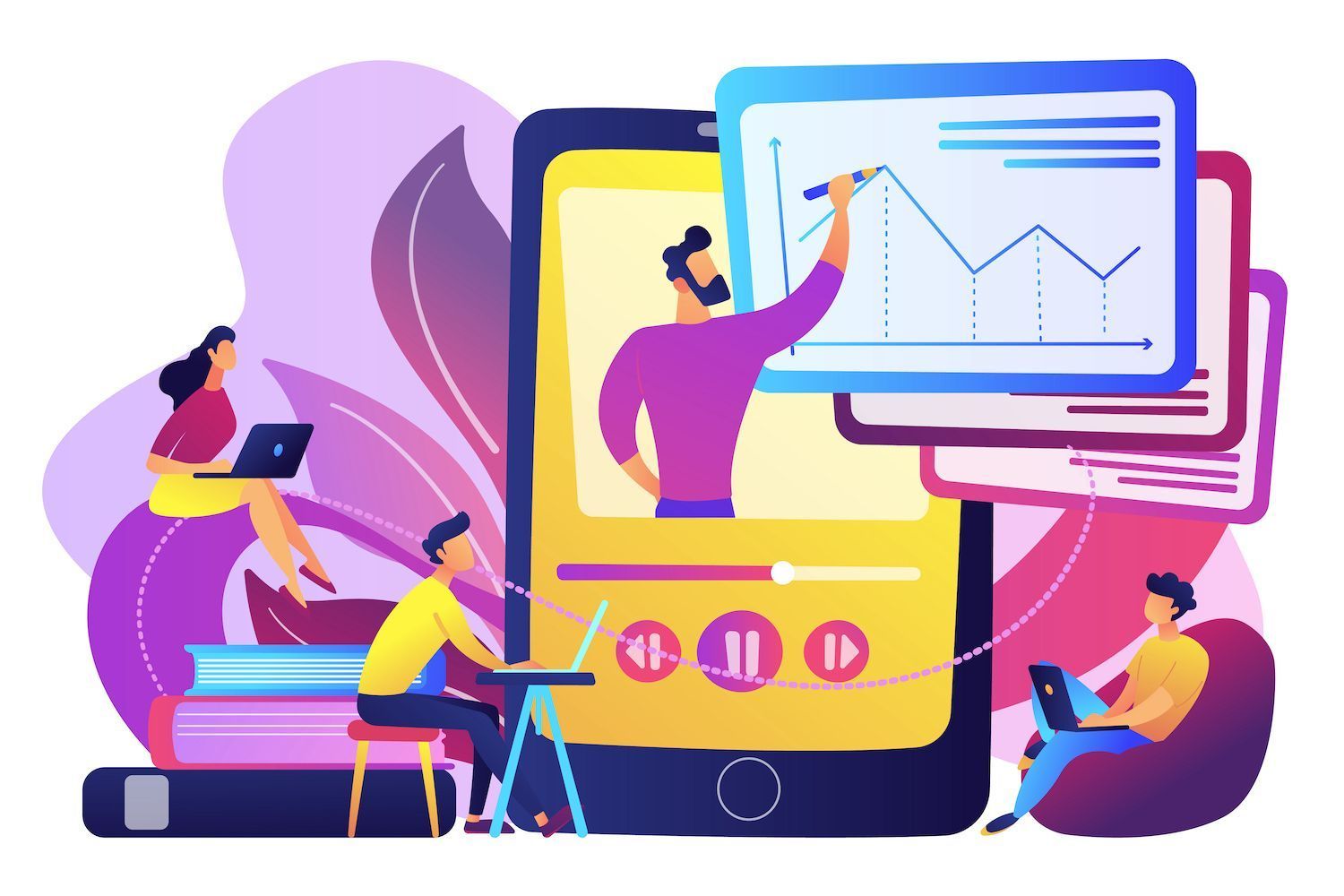
-sidebar-toc>
With cameras becoming more advanced and become more advanced, real-time object detection is becoming a well-known option. From autonomous vehicles to intelligent surveillance systems, to augmented reality applications the technology can be used in an array of applications.
Computer vision is an obscure term used to describe the method that uses computers and cameras to carry these kinds of operations. previously mentioned, is a huge and complicated area. Many people are unaware that it's possible to begin taking part in the immediate detection of objects using your web browser.
The circumstances
Here is a listing of principal technologies utilized for this piece:
- TensorFlow.js: TensorFlow.js is a JavaScript library that delivers the power of machine learning to the browser. It lets you download already trained models that have been trained to perform object recognition and then run them in the browser. It removes the need to perform complex server-side processing.
- Coco SSD The program uses an object recognition model which is trained and by the name of Coco SSD which is a light model that is capable of recognising the vast majority of common objects instantly. Although Coco SSD is a powerful tool, be aware that it is created using a whole set of objects. If you've got specific detection needs, you can build a custom model using TensorFlow.js using following this tutorial.
Begin a new React project
- Make a brand fresh React project. You can do this by following these commands:
NPM create vite@latest -object detection React template
This will generate an initial React project you are able to create using the vite.
- After that, then you can install TensorFlow along with The Coco SSD libraries by running these commands within the project:
npm i @tensorflow-models/coco-ssd @tensorflow/tfjs
Now is the time to begin developing your app.
Configuring the app
Before writing the code needed to implement the logic for object detection, let's look at the code that was created in this tutorial. The app's interface could be:
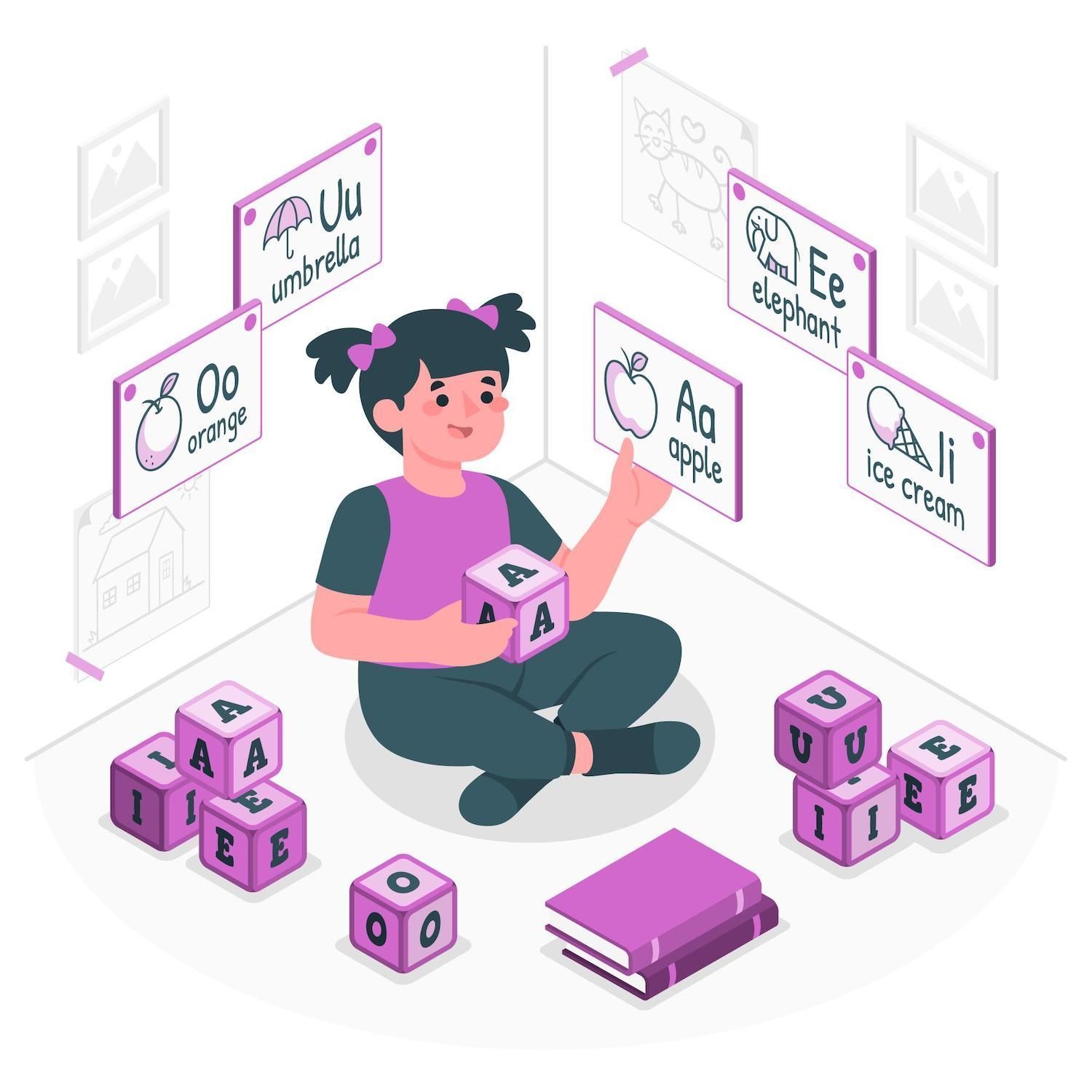
When users press the Start Webcam button when they click the Start Webcam button, they're prompted to grant permission for the app to use webcam feeds. Once permission has been granted, the app will showing the live feed from the webcam and recognizes the things it finds in the feed. After that, it creates an equilateral triangle to show the item it finds in the feed live and label them, and after that, it labels them.
In the initial step, develop a user-friendly interface of your app. Copy these steps into app.jsx. App.jsx file:
import ObjectDetection from './ObjectDetection'function App() return ( Image Object Detection ); Export default App
This code fragment creates the header of the page. It also integrates a customized component called "ObjectDetection"
. This component is responsible for taking a webcam's feed, and finding objects at a moment's notice.
In order to create this component create a brand-new document named ObjectDetection.jsx in your homedirectory and then copy the following code in it:
UseEffect and useRef as well as useState from'react'. Const objectDetection = () /const videoRef = useRef(null) Const [isWebcamStarted], setIsWebcamStarted] = useState(false) Const setWebcam to async () = // TODO; const stopWebcam = () => // TODO ; return ( isWebcamStarted? "Stop" : "Start" Webcam isWebcamStarted ? : ); ; export default ObjectDetection;
Here's how to use the code to create startWebcam. "startWebcam"
function:
const startWebcam = async () => try setIsWebcamStarted(true) const stream = await navigator.mediaDevices.getUserMedia( video: true ); if (videoRef.current) videoRef.current.srcObject = stream; catch (error) setIsWebcamStarted(false) console.error('Error accessing webcam:', error); ;
The system will request users to give access rights to their web camera and when granted permission it will modify video. video
to show the live feed of their webcam for the person using the service.
If the program is unable to access the camera feed (possibly due to the absence of a webcam installed on the device or a reason why the user has not been granted access) it will display an error message to the console. The console can print an error message that explains what caused the problem to the user.
The next step is to replace the stopWebcam
function with this code:
const stopWebcam = () => const video = videoRef.current; if (video) const stream = video.srcObject; const tracks = stream.getTracks(); tracks.forEach((track) => track.stop(); ); video.srcObject = null; setPredictions([]) setIsWebcamStarted(false) ;
The code looks for any video streams that can be accessed through the video
object and stops every one of the streams. Then, it changes the isWebcamStarted
status to True
.
In this instance, you can try running the app to check if you can access and see the feed on the webcam.
It is necessary to paste the code within your index.css file to ensure that your application looks exactly like the one you have seen earlier:
#root font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif; line-height: 1.5; font-weight: 400; color-scheme: light dark; color: rgba(255, 255, 255, 0.87); background-color: #242424; min-width: 100vw; min-height: 100vh; font-synthesis: none; text-rendering: optimizeLegibility; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; a font-weight: 500; color: #646cff; text-decoration: inherit; a:hover color: #535bf2; body margin: 0; display: flex; place-items: center; min-width: 100vw; min-height: 100vh; h1 font-size: 3.2em; line-height: 1.1; button border-radius: 8px; border: 1px solid transparent; padding: 0.6em 1.2em; font-size: 1em; font-weight: 500; font-family: inherit; background-color: #1a1a1a; cursor: pointer; transition: border-color 0.25s; button:hover border-color: #646cff; button:focus, button:focus-visible outline: 4px auto -webkit-focus-ring-color; @media (prefers-color-scheme: light) :root color: #213547; background-color: #ffffff; a:hover color: #747bff; button background-color: #f9f9f9; .app width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: column; .object-detection width: 100%; display: flex; flex-direction: column; align-items: center; justify-content: center; .buttons width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: row; button margin: 2px; div margin: 4px;
You must delete the App.css file to ensure that you don't mess up the style of your elements. You are now ready to apply the required logic of real-time object recognition into your app.
Set up real-time object detection
- The initial step is to add the imports from Tensorflow as well as Coco SSD at the top of ObjectDetection.jsx :
import * as cocoSsd from '@tensorflow-models/coco-ssd'; import '@tensorflow/tfjs';
- Make a condition new in the
ObjectDetection
component, in order to save the prediction array generated by the Coco SSD model:
const [predictions setPredictions, useStatesetPredictions, useState ([]);
- Then, you can create a program to load in Coco SSD model. Then it loads onto the Coco SSD model, collects footage and then makes a prediction:
const predictObject = async () => const model = await cocoSsd.load(); model.detect(videoRef.current).then((predictions) => setPredictions(predictions); ) .catch(err => console.error(err) ); ;
This program makes use of the video feed to make the predictions for objects in the feed. The program provides you with an assortment of objects predicted to appear with each one having a tag with a percentage of the confidence level and numbers which indicate the position of the object within the video's frame.
It is essential to invoke this feature to process videos as frames are added and then use the forecasts that are saved in the predictions
state to display boxes and labels for every known object in the video stream that is live.
- After that, you'll be able to use the
the setInterval
function that calls this method on a frequent schedule. Also, you must make sure that this function is not active after the user is shut off from the feed from their webcam. In order to prevent this from taking place, you must use theClearInterval
functionality in JavaScript.Add the container for state and useEffect hooks theeffects
hooks within theelement object detection
element in order to create thepredictObject
function, which runs indefinitely when the webcam is turned on and then removed from the websitecam when it's shut off.
const [detectionInterval, setDetectionInterval] = useState() useEffect(() => if (isWebcamStarted) setDetectionInterval(setInterval(predictObject, 500)) else if (detectionInterval) clearInterval(detectionInterval) setDetectionInterval(null) , [isWebcamStarted])
The app is set up to identify objects in the view of the camera every 500 milliseconds. It is possible to alter the number of milliseconds depending upon the rate you'd like the object detection speed to occur, but be aware of the possibility of using it too frequently. can result in your application making use of a significant amount of memory in the browser.
- When you've got the data for your prediction then you're able to utilize the information to formulate predictions. When you've got a prediction, it can be used to state containers. It is possible to utilize it to display the label and also it as a container in the live feed of your video. For this to happen it is necessary to change your
returning
declaration of theobject detection
Input the following info:
Return ( isWebcamStarted ? "Stop" : "Start" Webcam isWebcamStarted ? : /* Add the tags below to show a label using the p element and a box using the div element */ predictions.length > 0 && ( predictions.map(prediction => return prediction.class + ' - with ' + Math.round(parseFloat(prediction.score) * 100) + '% confidence. ' > ) ) /* Add the tags below to show a list of predictions to user */ predictions.length > 0 && ( Predictions: predictions.map((prediction, index) => ( `$prediction.class ($(prediction.score * 100).toFixed(2)%)` )) ) );
The program will display the prediction list beneath the feed from the webcam. The program then creates an area around the forecasted object with the coordinates for Coco SSD as well as names in the middle of the boxes.
- For styling the boxes and labels properly, insert this code into index.css File: index.css file:
.feed position: relative; p position: absolute; padding: 5px; background-color: rgba(255, 111, 0, 0.85); color: #FFF; border: 1px dashed rgba(255, 255, 255, 0.7); z-index: 2; font-size: 12px; margin: 0; .marker background: rgba(0, 255, 0, 0.25); border: 1px dashed #fff; z-index: 1; position: absolute;
The application is completed. the application. The application is now able to restart the development server to test the software. What happens when the program is complete
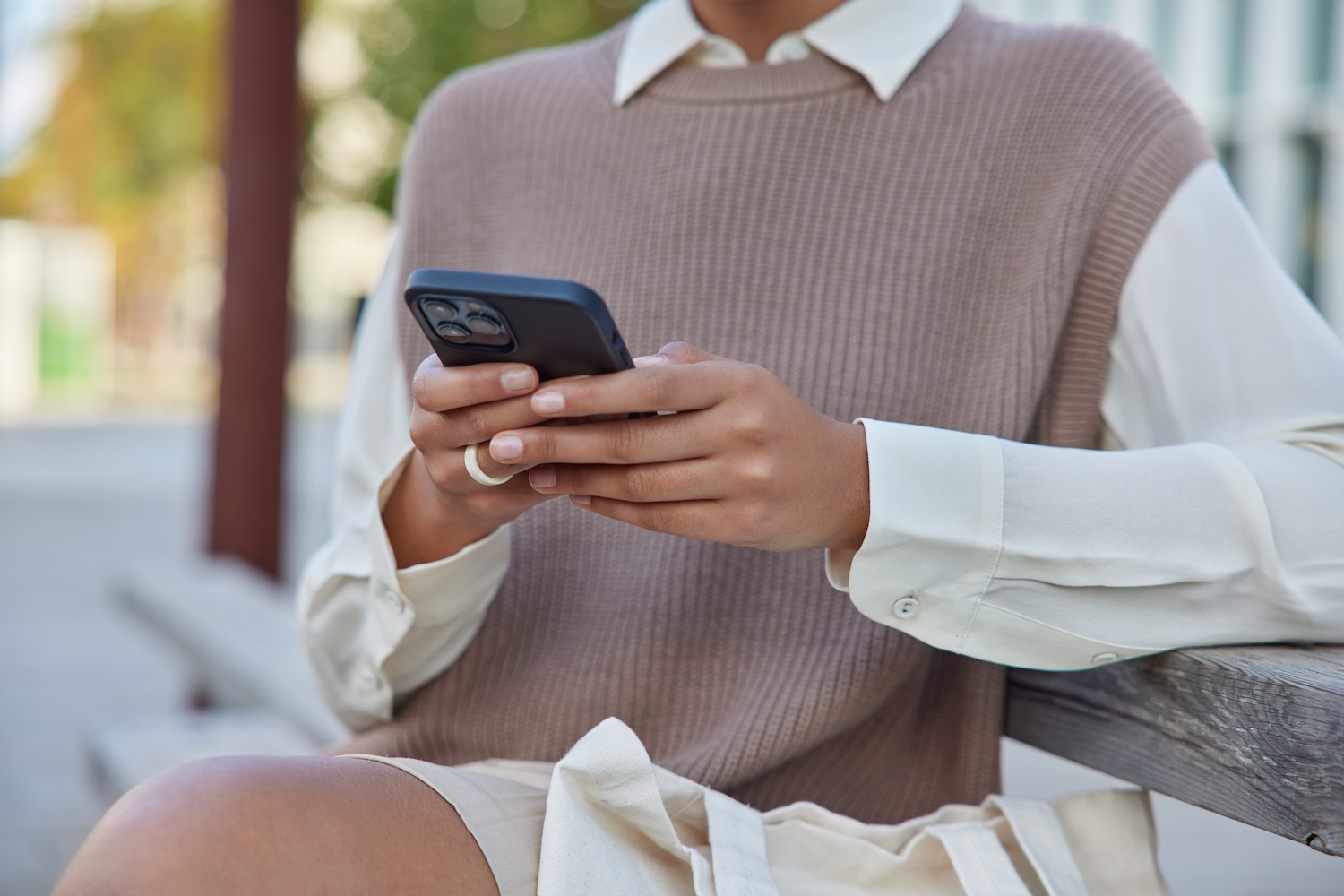
Complete code is available is available in the repository at GitHub. GitHub repository.
Deploy the completed app to
Once your repository on Git is operational then follow these steps to install the app on :
- Log in or create an account to see the dashboard of Your Dashboard. My dashboard.
- Authorize with your Git provider.
- Select the static sites on the sidebar to the left and choose Add Site. Choose Add Site.
- Select the branch and repository you wish to have be able to access via.
- Assign a unique name to your site.
- Incorporate the settings for building in accordance with the following format:
- Build command:
yarn build
orNPM run build
- Node version:
20.2.0
- Publish directory:
dist
- Then, click Create site.
Once the app is launched, click "Visit website" from the dashboard in order to open the application. You can test the app using different devices with cameras and see what happens.
Summary
It's been successful to develop an object detection in real-time and live time application using React, TensorFlow.js, and . It allows you to explore the potential of computer vision, and create interactive experiences in the user's browser.
Be aware that our Coco SSD model we used is only a starting base. If you'd like to continue looking into the options, consider being able to customize the detection of objects with TensorFlow.js that allows you to tailor the application to find the best objects to meet the specific needs of your organization.
The possibilities are endless! The app can be the basis for developing advanced applications like Augmented Reality Experiences along with advanced surveillance techniques. If you launch your app via the secure platform, you are able to share your work with everyone all over the world, and see the possibilities of computer vision come to life.
What's the biggest issue you've encountered which you think the real-time detection of objects could help be able to solve? Comment on your experiences in the comments below!
Kumar Harsh
Kumar is a developer of technical software as well as a writer with a base in India. He's an expert in JavaScript in addition to DevOps. Learn more about his expertise on his website.
This article was originally posted this site.
Article was posted on here