How do you create and use fake records using the Model Factories in Laravel - (r)
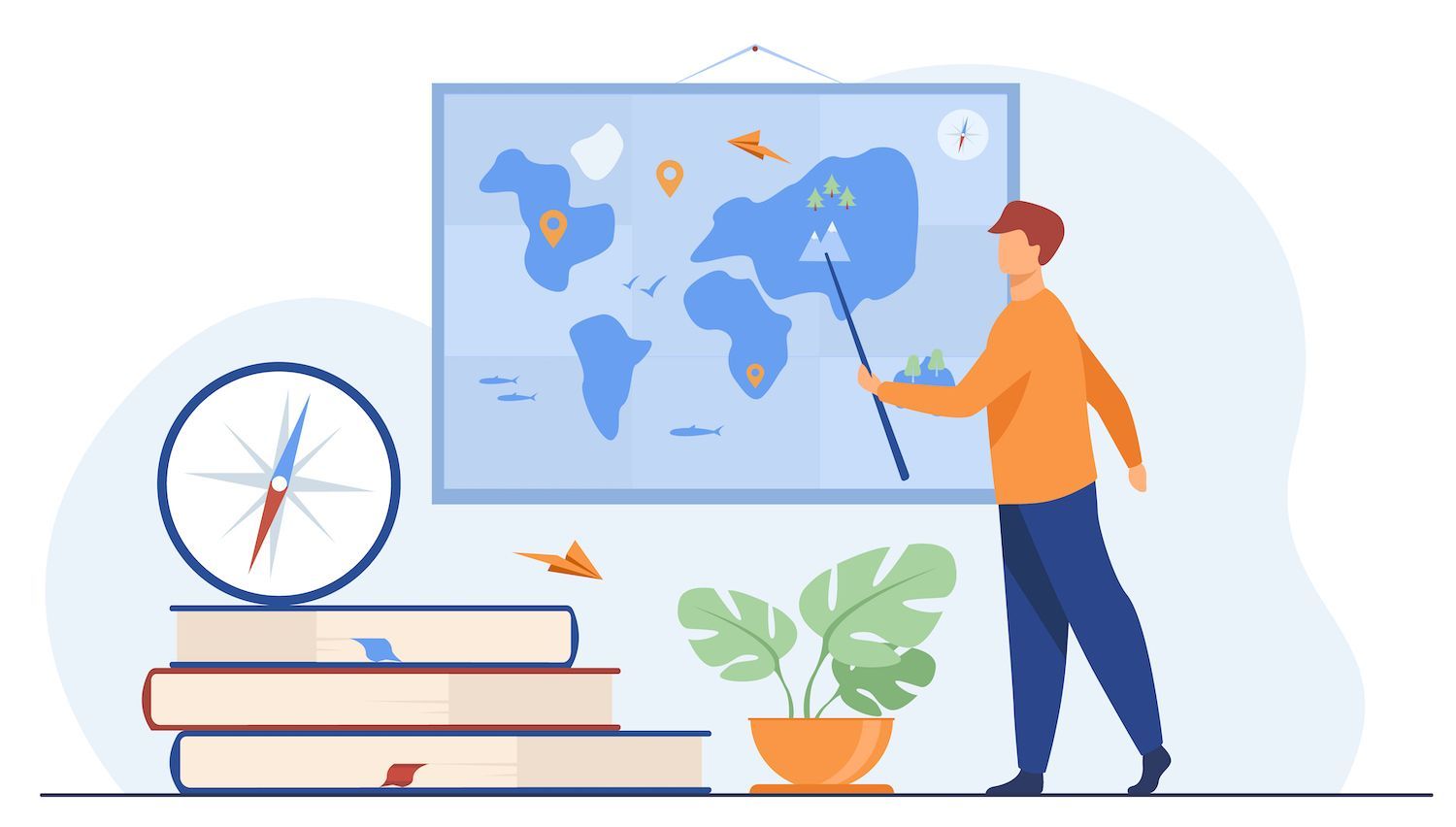
Please share the news with
In the event of creating an online blogging platform that permits authors and moderators to review comments prior to when they go live, you'd need to test if it functions properly prior to deploying it to your customers. The test needs details.
This article explains how to get comments data without any real user comments.
The prerequisites
In order to complete this tutorial, it is essential to know these subjects:
- XAMPP
- Composer
Download the complete code for the project, so you are able to follow the project.
How To Set Up the Project
In this this section, you'll build a Laravel project, and then link it up to an online database. In this section, we'll discuss what it takes and the steps to accomplish this.
Install Laravel Installer
To build a Laravel project fast, install Laravel's installer. Laravel installer.
composer global require laravel/installer
This code will install the Laravel installer on a global basis. device.
Develop the Laravel Project
laravel new app-name
The code bootstraps a brand fresh Laravel project, and then installs all dependencies:
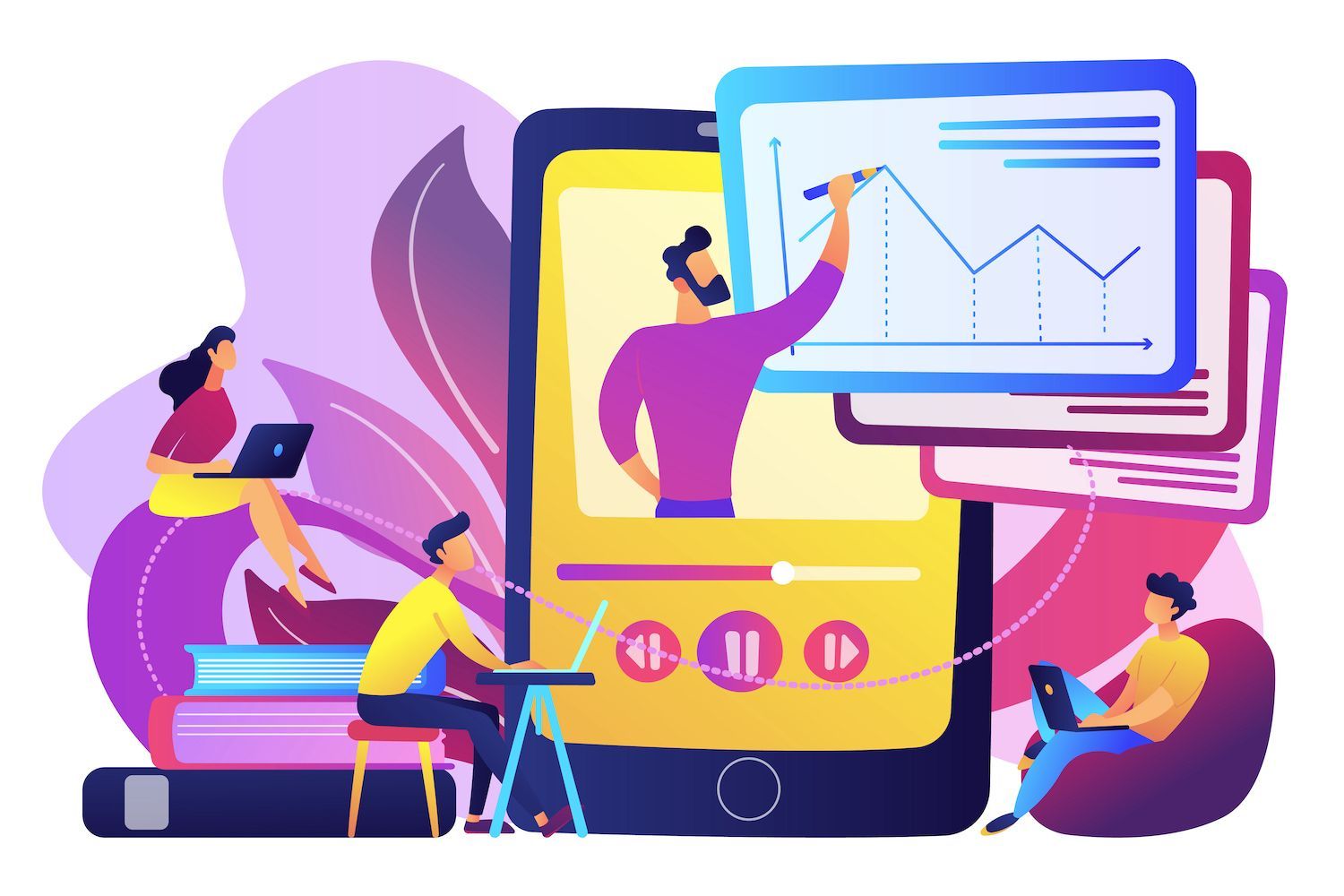
Another easier way to install Laravel is to take advantage of Composer direct.
composer create-project laravel/laravel app-name
It is not necessary to download the Laravel installer. Installer for Laravel is available if you follow the procedure above.
Launch the app
The ability to change the directory's application name and start the project using Laravel's own Command-Line Interface (CLI) tool, Artisan:
php artisan serve
The code starts the project by connecting to localhost:8000 or any other port that is open, when port 8000 is utilized. When you connect to localhost:8000, you will find the following:
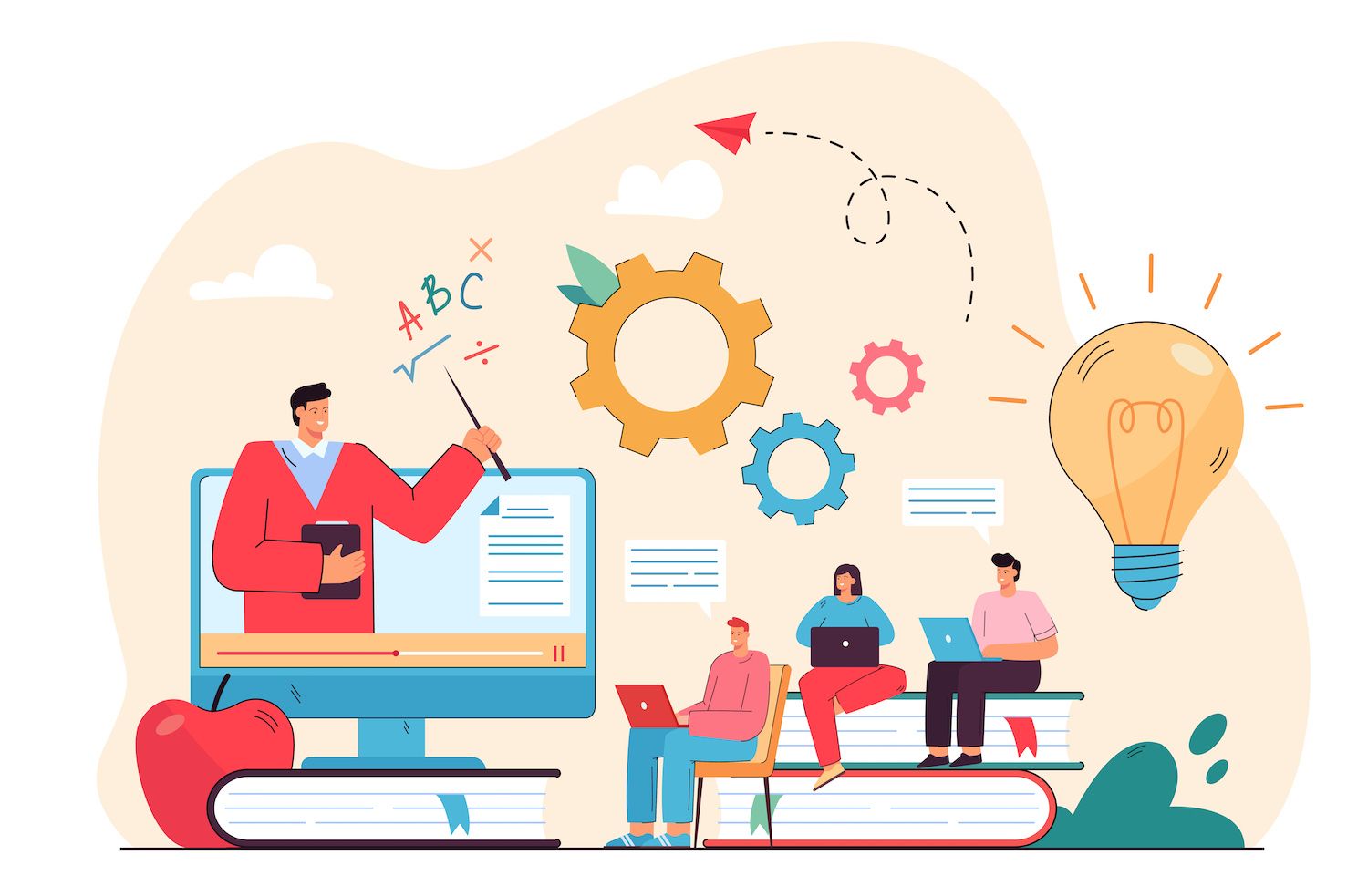
Create an Database
To connect your app to a database, you must build a new database using the XAMPP PHPMyAdmin GUI. Go to http://localhost/phpmyadmin and select New on the sidebar:
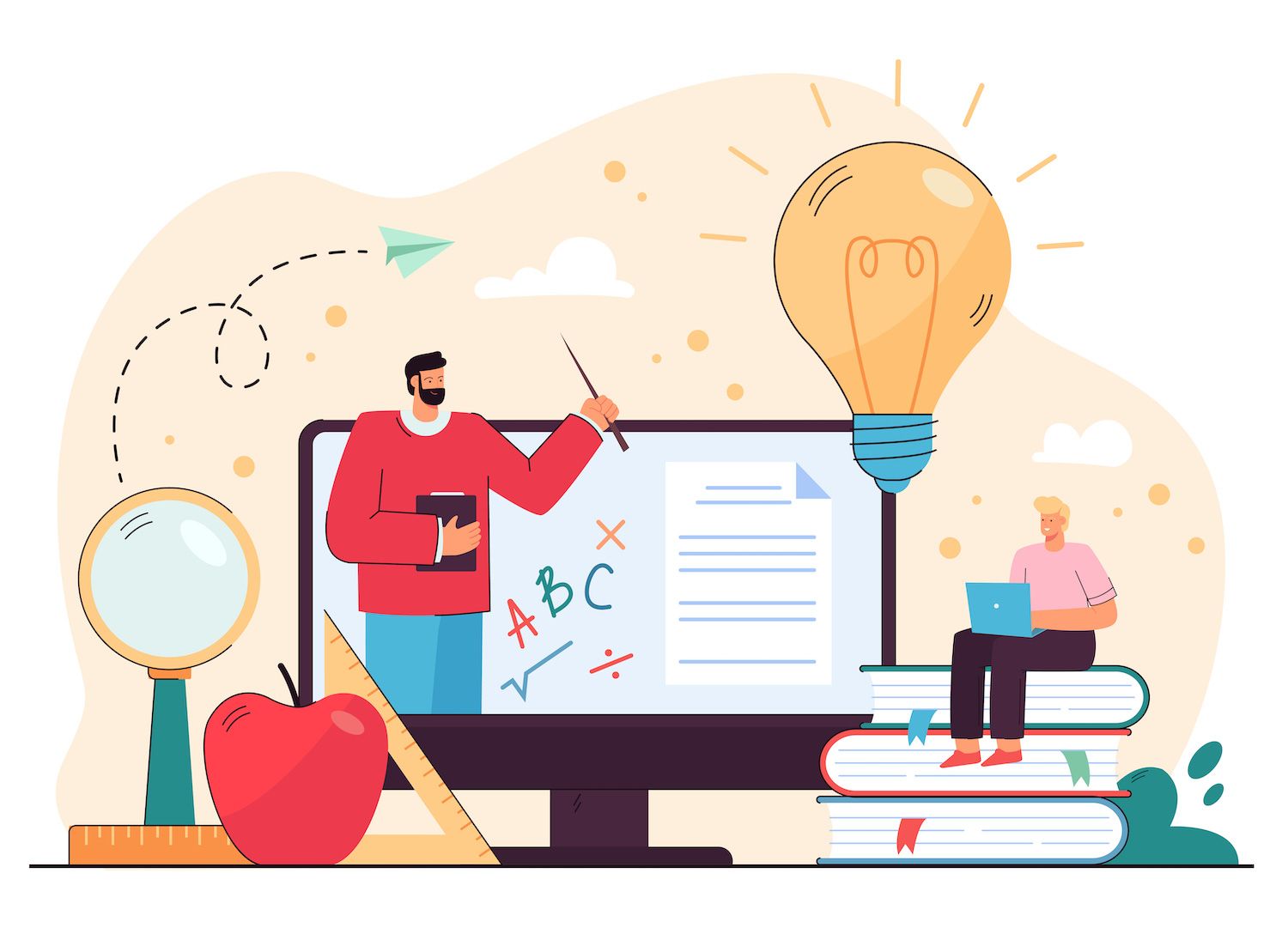
This picture shows you how to fill out the Create Database form which uses App_name as the name for the database.
Click "Create" to build a database of your account.
Modify the .env Modify the.env file
In order to connect your application with your database you will need to modify the DB section of your .env file:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=app_name DB_USERNAME=root DB_PASSWORD=
The code will fill in the data in your database using the database's name username port, password and host. You're now ready to start creating factories and models.
Note: Replace the values with your login credentials for your database. If you encounter the "Access refused for user" error, ensure you put the data to the DB_USERNAME
as well as the DB_PASSWORD
with double quotation mark.
How to Generate Fake Data
After creating the app and connecting it with your database will then create the files for creating false data in the database.
Make the Comment Model
The model file is created to interact with the databases tables. To create a model, use Artisan:
php artisan make:model Comment
This code creates a Comment.php file inside the application/M orels folder. It contains boilerplate code. The following code should be added below the Use HasFactory
line:
protected $fillable = [ 'name', 'email', 'body', 'approved', 'likes' ];
Below is a list of fields you'd like to be able to accept massive assignments because Laravel secures your database against massive assignments automatically. The Comment model file should now appear like:
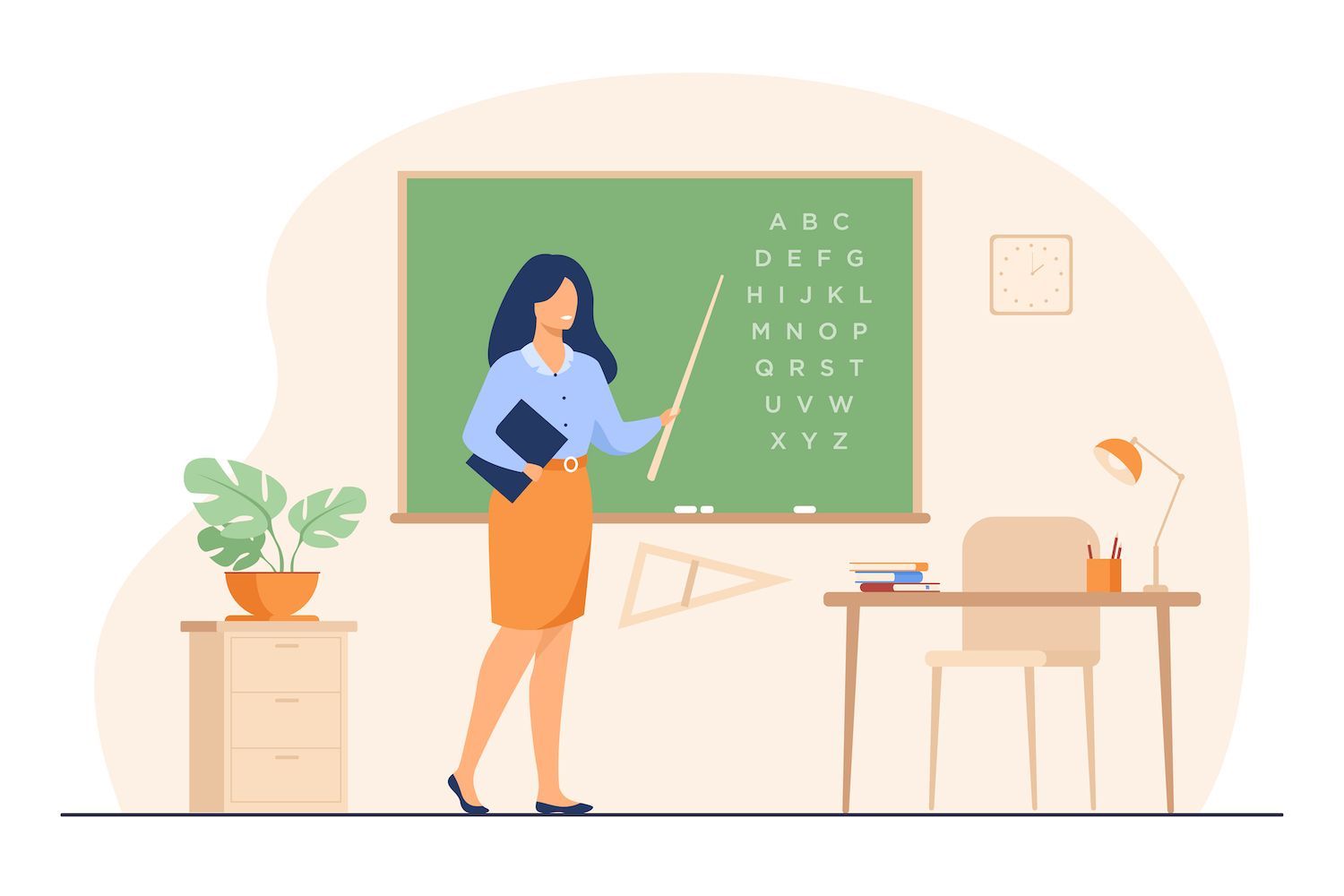
Create the Migration File
After you've created your model file, after declaring the $fillable
array, you will be required to build the migration file in the manner described below:
php artisan make:migration create_comments_table
Notice: The naming convention used to build migrations within Laravel generally known as the snake_case
that is referred to as underscore_case
. The first word refers to the process, while the second is the plural form of the model. The final word refers to the function that gets created inside the program. That's why you enter create_books_table
in the process of creating a migration for the Book model.
This code creates a file named yyyy_mm_dd_hhmmss_create_comments_table inside the database/migrations folder.
Next, edit the up function inside yyyy_mm_dd_hhmmss_create_comments_table:
public function up() Schema::create('comments', function (Blueprint $table) $table->id(); $table->string('name'); $table->string('email'); $table->longText('body'); $table->boolean('approved'); $table->integer('likes')->default(0); $table->timestamps(); );
The code generates the schema that will allow for the columns, name, email body, approval, likes, and timestamps.
Run the Migrations
Making and editing the files for migration won't achieve any good until you execute them using on the command line. When you visit the Database Manager, you'll find that the database manager is empty.
Do the migrations using Artisan:
php artisan migrate
The command will run all updates in the databases/migrations since it's the first time a migration has been run since the program was launched:
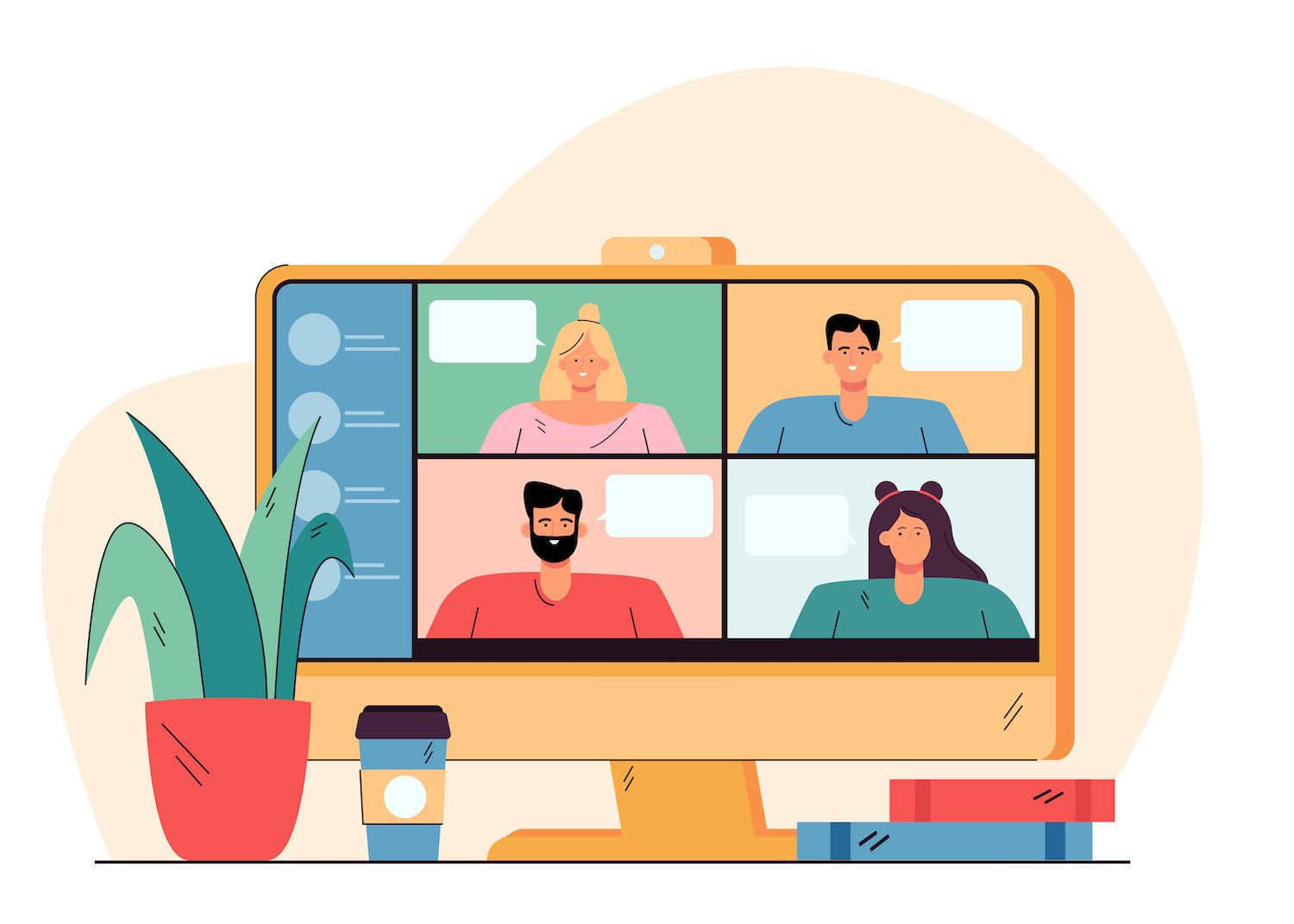
Below, you can see all the migration files that you have executed. Each file is a table of the database:
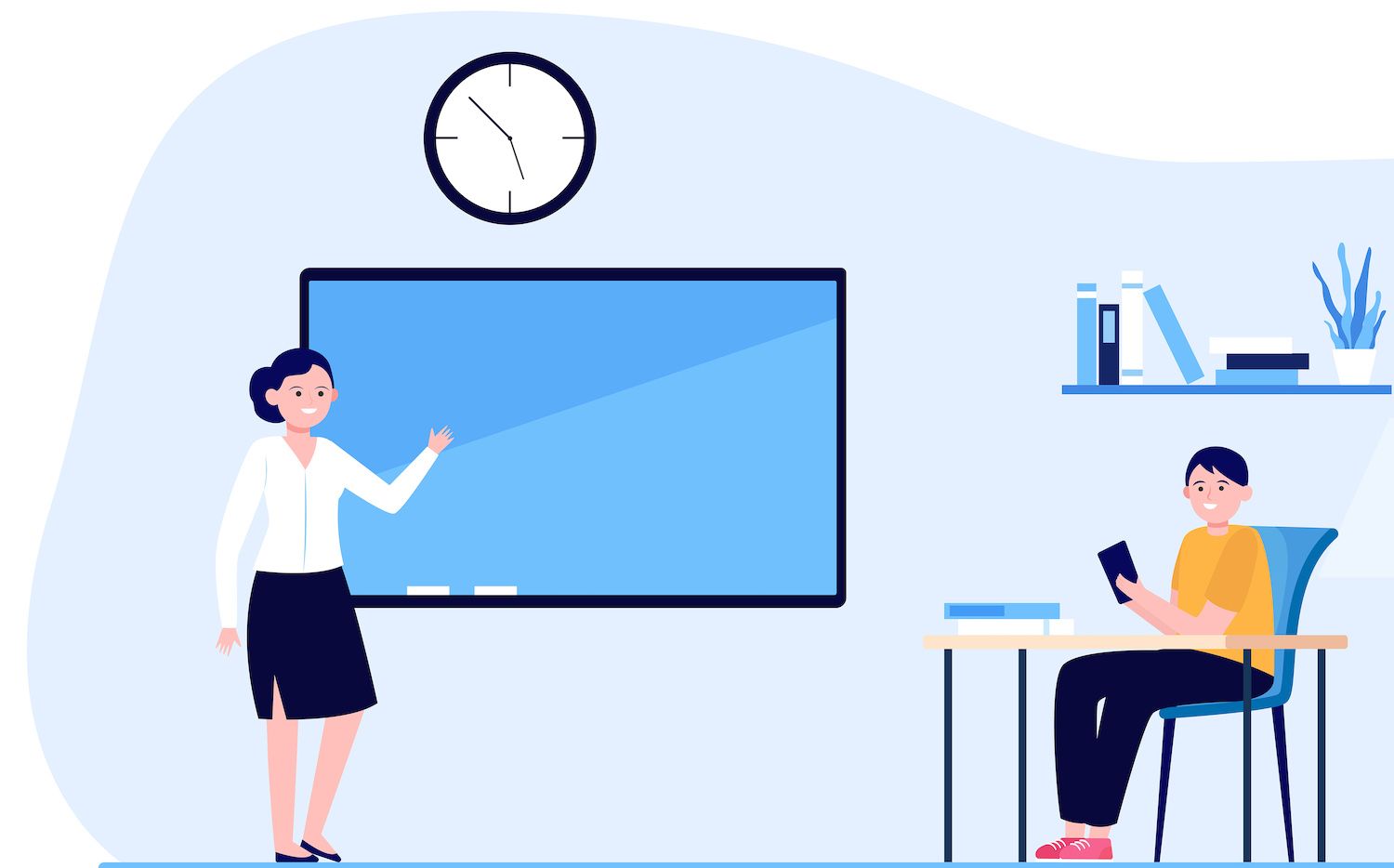
Create the CommentFactory File
Make a factory with the definition function. In this course, you'll build a factory with Artisan:
php artisan make:factory CommentFactory.php
The code generates a CommentFactory .php file in the directory database/factories directory.
Definitions and their Function Definition
The process that is described in CommentFactory shows the manner in which Faker generates false information. Edit it to look as follows:
public function definition() return [ 'name' => $this->faker->name(), 'email' => $this->faker->email(), 'body' => $this->faker->sentence(45), 'approved' => $this->faker->boolean(), 'likes' => $this->faker->randomNumber(5) ];
This code tells Faker to produce the following:
- The name
- An email address
- A paragraph that has 45 sentences
- An authentic value that is only valid if the value is real or not.
- Random numbers that vary between zero and 9999.
The Comment Model can be connected to Comment Model to Comment Model to CommentFactory
Connect to the comment
model to the CommentFactory
by declaring a protected $model
variable over the definition of:
protected $model = Comment::class;
Additionally, you should also add ModelsComment, an app that you have installed
to the dependencies of your file. CommentFactory's file should now look like: CommentFactory file should now look like:
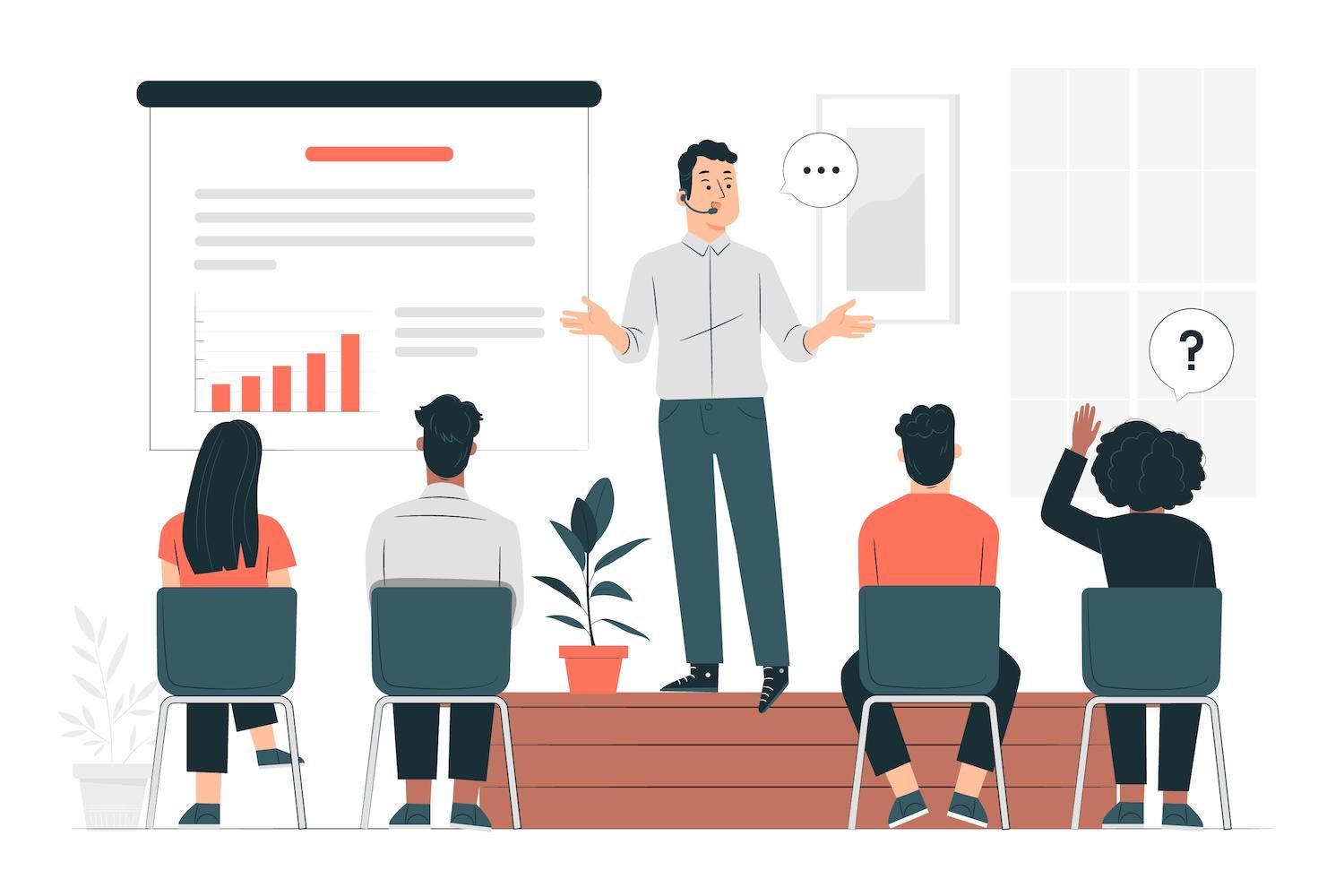
Steps to Begin Seeding the Database
In programming, seeding is the act of generating random data that can be stored in databases for test purposes.
Now that you've created the model, you can run it with changes, and then add the definition inside CommentFactory. You can run the database seeder with it's DatabaseSeeder file.
Make the CommentSeeder File
Create a seeder file, which uses factory information to create it:
php artisan make:seeder CommentSeeder.php
The code generates commentseeder.php, which is the CommentSeeder .php file within the database/seeders folder.
Modify the run function so that it can edit
Connect the comment model with the commentSeeder. Incorporate the following code into your run program:
Comment::factory()->count(50)->create();
This code tells the CommentSeeder to utilize the Comment model as well as the CommentFactory's definition function to generate 50 comments within the database. Add the appmodelsComment
to the dependency list of file. CommentsSeeder's file will now appear like this: CommentSeeder file will now appear like this:
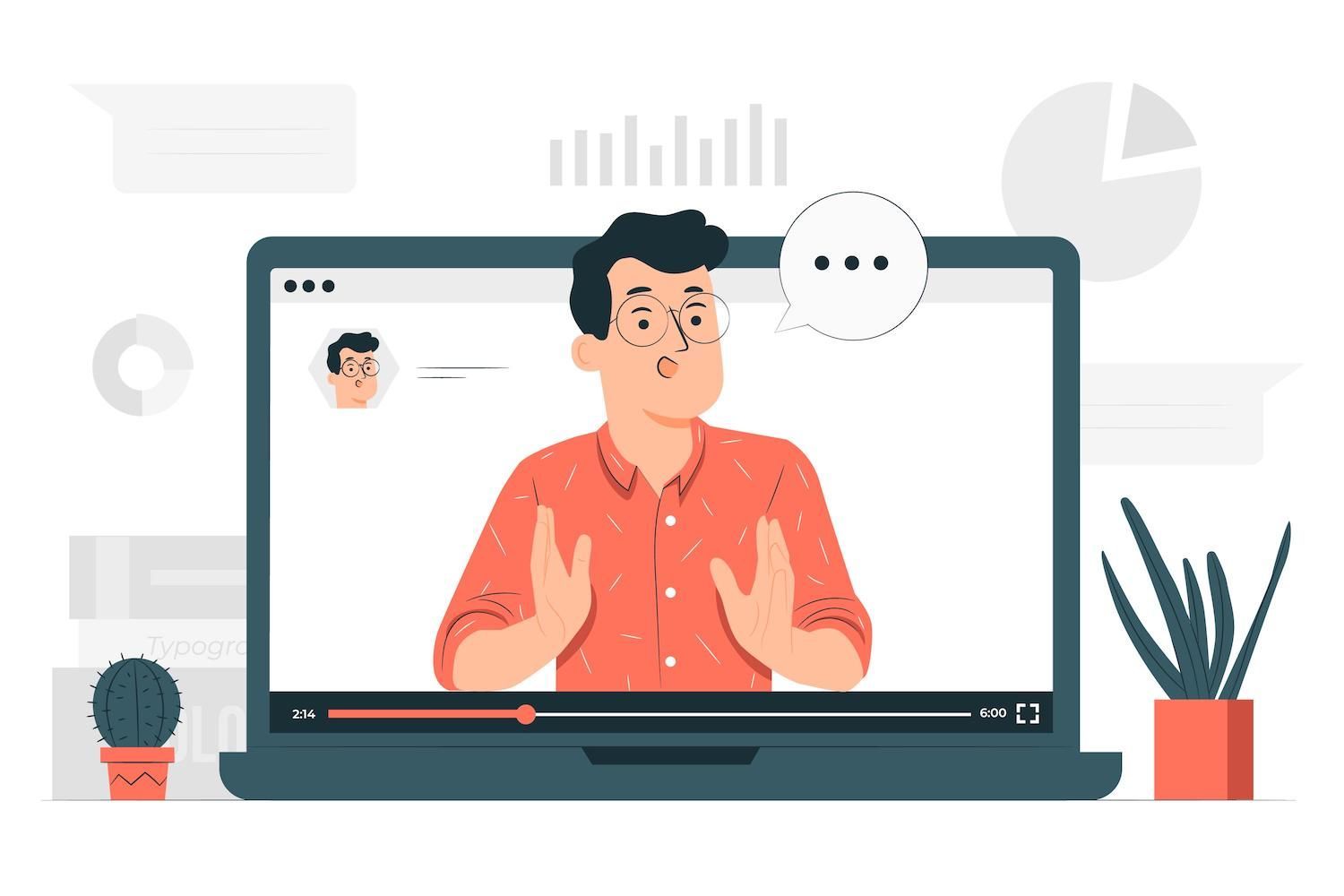
Note: You can configure Faker to create local information. You can, for instance, configure it to create Italian names, instead of random names by altering it's faker_locale
in the app/config.php file to it_IT
. For more information, read about Faker Locales in this instructional video.
Start the Seeder
After that, you'll be able to run your file to create a seeder application by using Artisan:
php artisan db:seed --class=CommentSeeder
The program executes on the Seeder file and produces 50 rows of fake data in the database.
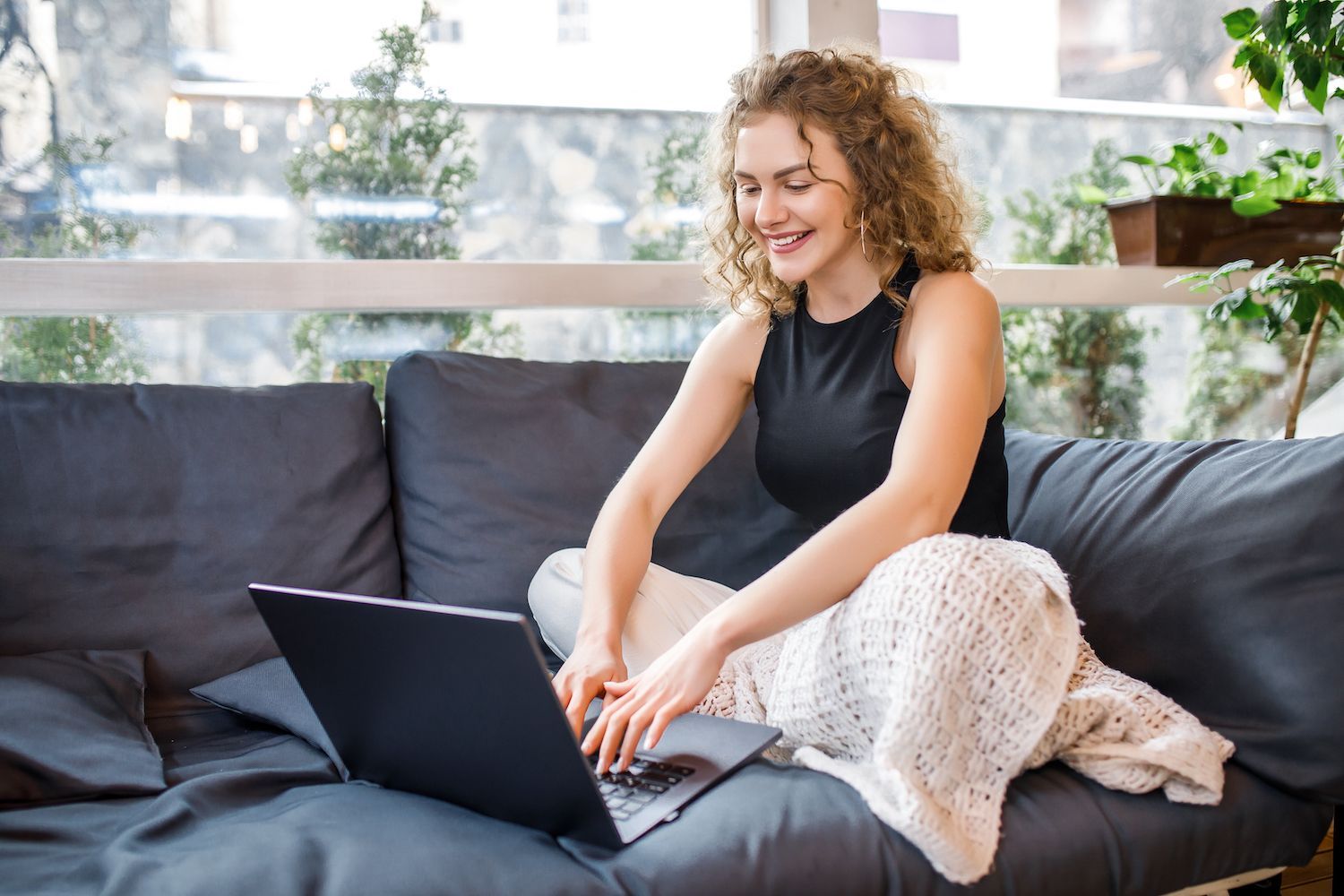
The database will now contain 50 rows of false information which you could use to check the functions of your program:
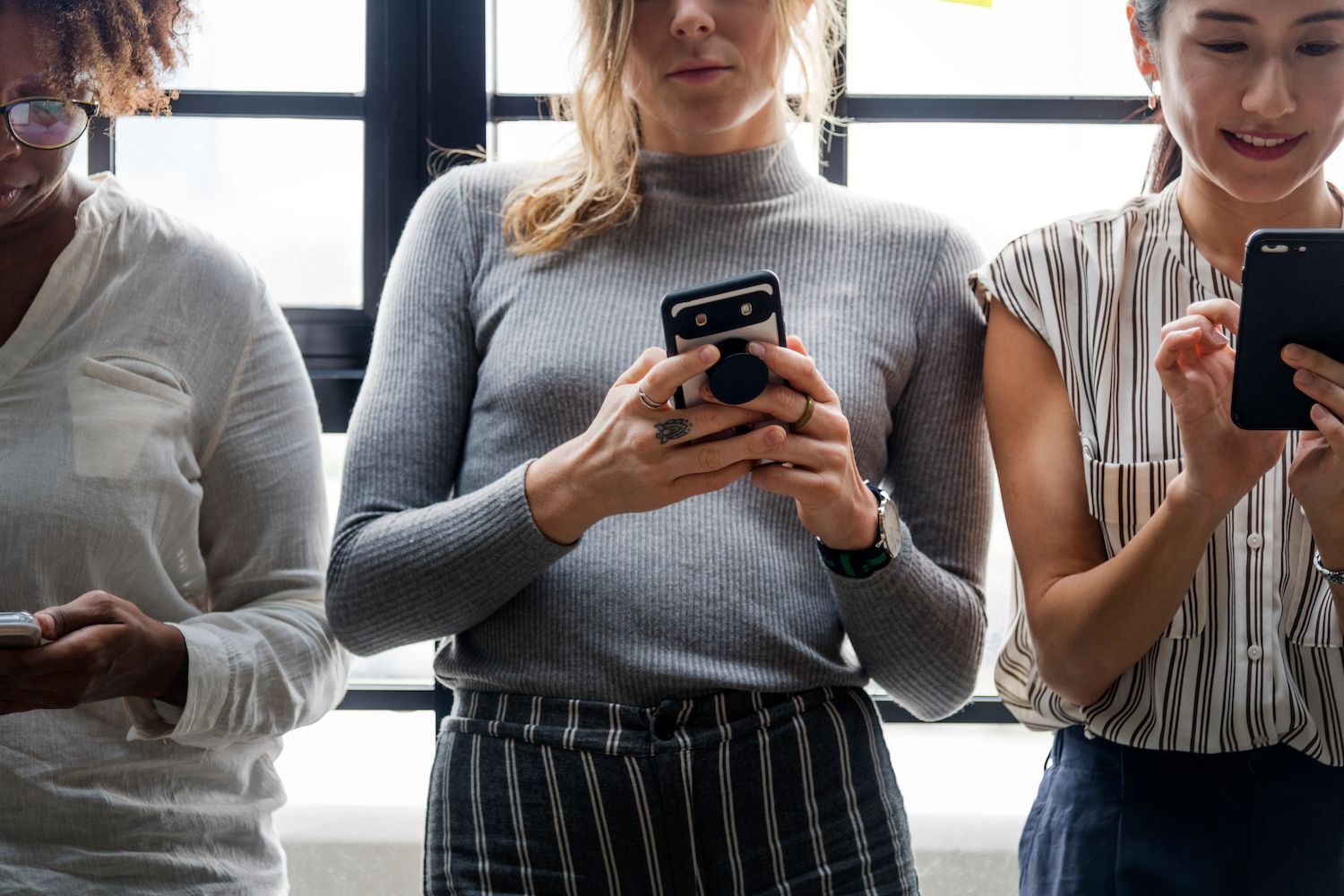
How To Reset the Database
If you are using generated information for testing, you may change your database each time you conduct a test. Suppose you wanted to test the feature of turning off comments that is approved. Refresh the database after every test to ensure the data that was generated previously isn't causing problems during the future tests.
Utilize RefreshDatabase
Make the database refreshed using the RefreshDatabase
trait inside the test file.
Navigate to ExampleTest.php inside the tests/Feature folder to the comment use Illuminate\Foundation\Testing\RefreshDatabase;
and add the following line of code above the test_the_application_returns_a_successful_response
function:
Make use of RefreshDatabase
The ExampleTest.php file should appear to look something similar to:
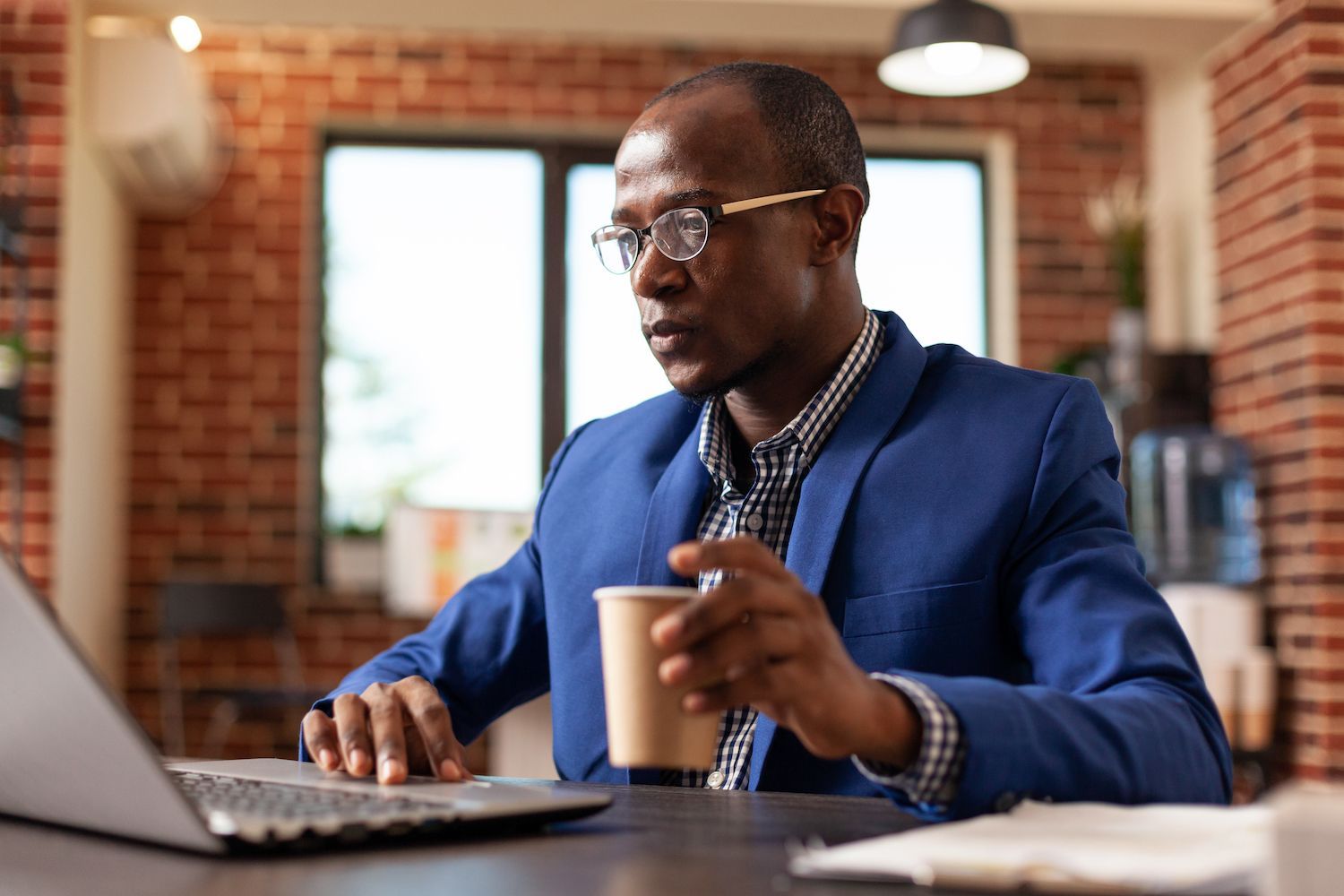
Do the test
Once you have added the RefreshDatabase
trait to the test file, execute the test using Artisan:
php artisan test
This code performs all tests within the app, and it will also refresh databases after each of tests being completed, as seen in the following image:
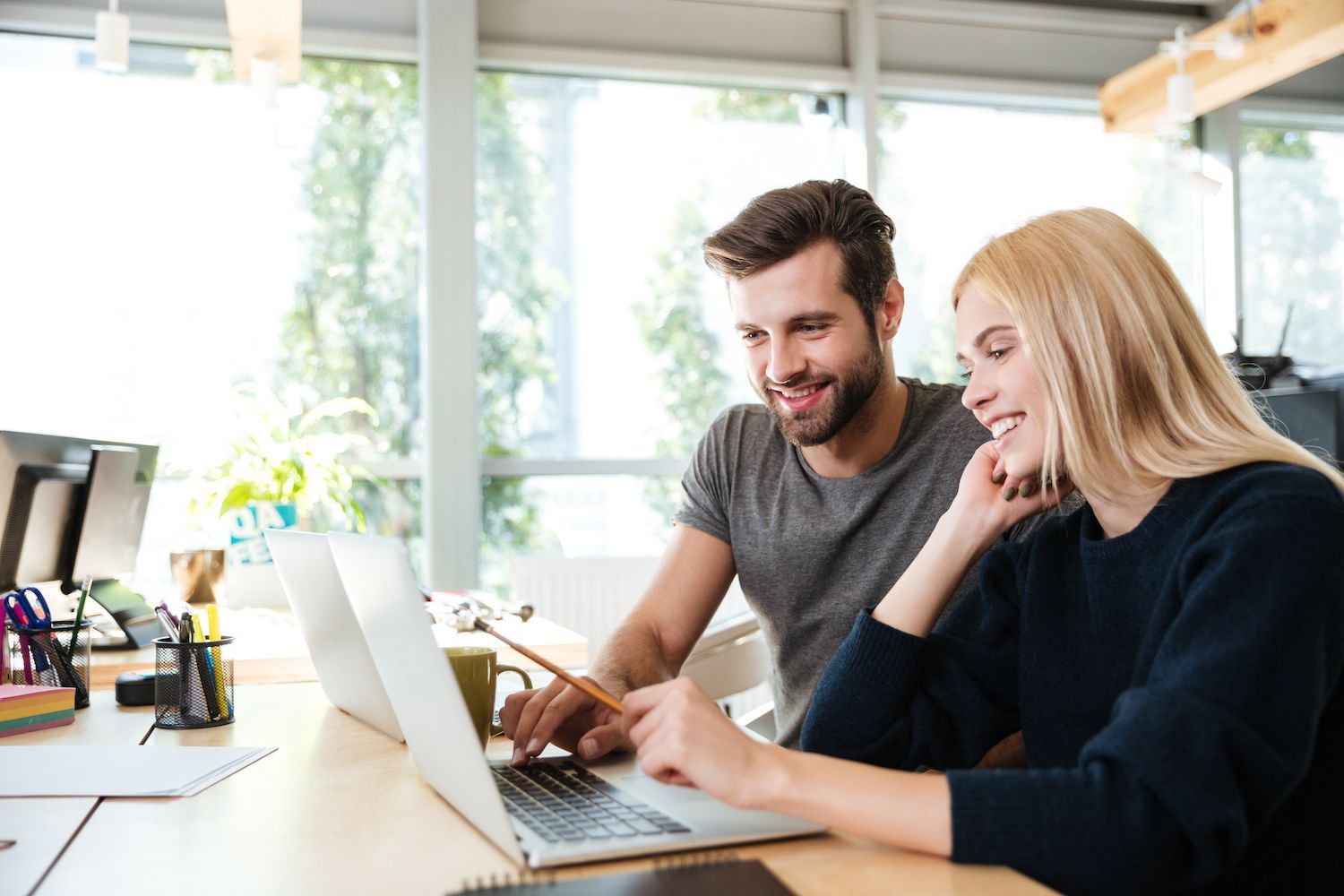
Now, check your database for the comments table empty:
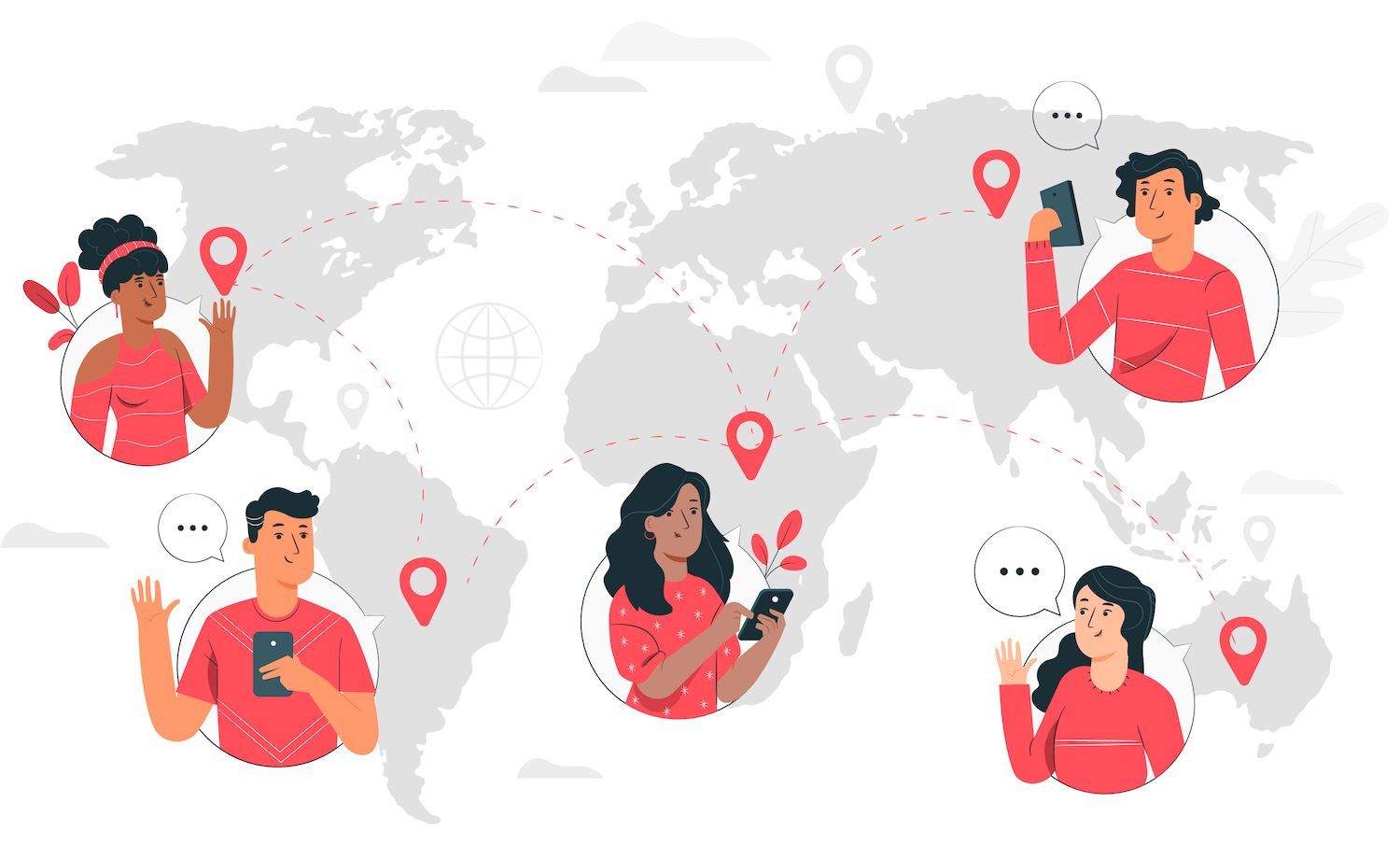
Summary
You've now seen how Laravel Factories and Faker make it possible to produce the whole amount of test data in minutes to test an application or even as placeholder data, with just a little setting up.
- Easy setup and management in My dashboard. My dashboard
- 24 hour expert assistance
- The top Google Cloud Platform hardware and network driven by Kubernetes to provide the highest performance and scalability
- A high-end Cloudflare integration to speed up the process and increase security
- Reaching a global audience with up to 35 data centers, and more than 275 PoPs across the globe
This post was first seen on here