This is Building of GraphQL APIs Using Node
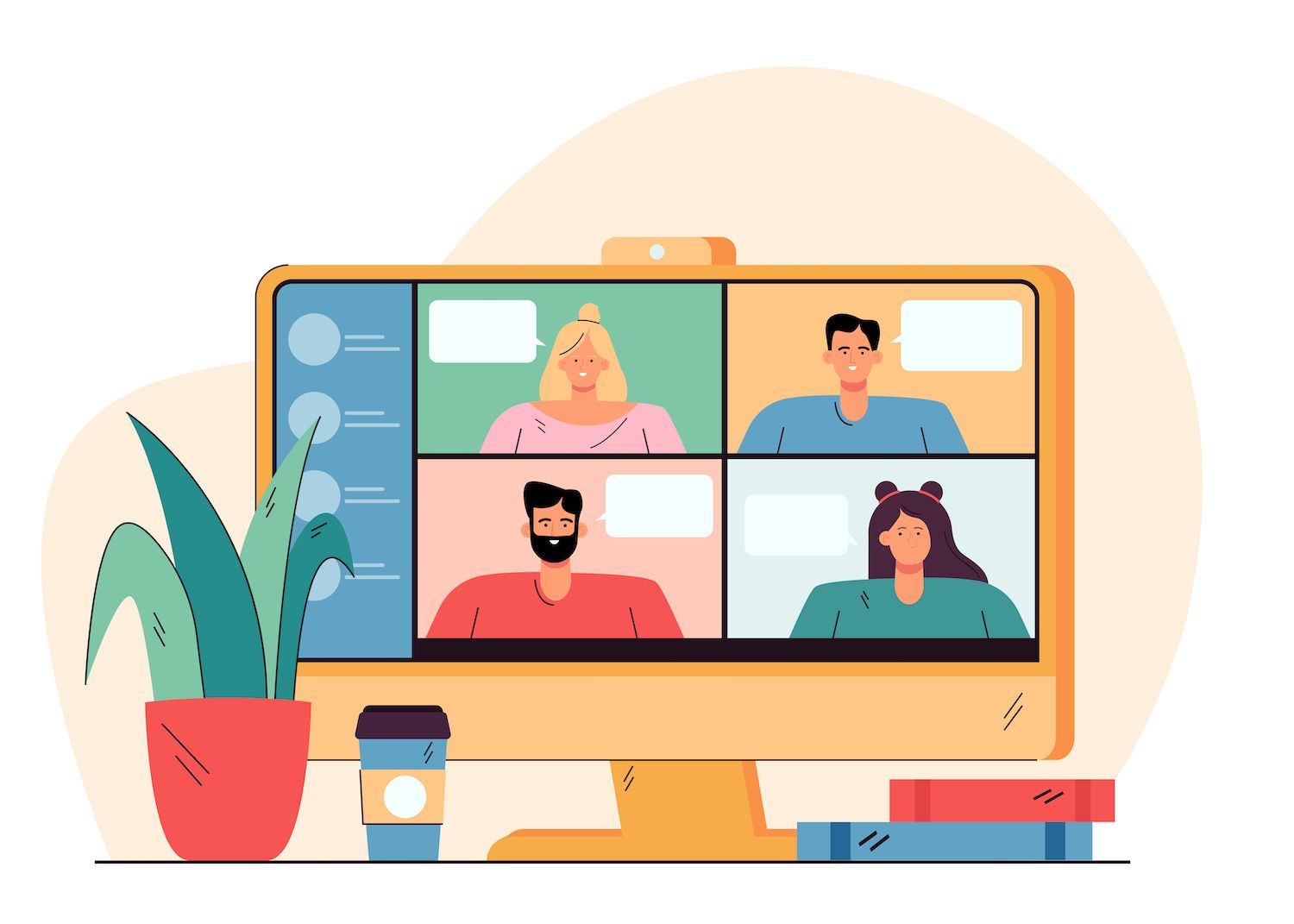
GraphQL is the latest technology used to develop APIs. Though RESTful APIs have been the method that is most commonly used to display data to apps, they have their limitations that GraphQL aims to solve.
GraphQL is the query language developed by Facebook which was transformed into an open-source project in the year of 2015. T provides a user-friendly and flexible syntax to explain as well as accessing information via an API.
What exactly is GraphQL?
According to the official info: "GraphQL is a API query language and an API runtime that can be used to complete queries using your existing information. GraphQL offers a comprehensive and simple explanation of the information that you provide through your API. It allows clients to get exactly what they need and no more. This allows it to be easy to build APIs within a short the time available and it also gives developers with powerful tools."
For creating an GraphQL service, begin with defining schema types before developing fields based on those kinds. You then create the function resolver which is run on each type and field whenever information is needed on the client's side.
GraphQL Terminology
GraphQL type system is utilized to determine what information can be requested as well as what data is possible to alter. This is the core of GraphQL. We'll look at various ways in which we could describe and modify the data in the framework of GraphQ.
Types
GraphQL objects are models that contain fields that have a type of strong. It is suggested to create an 1-to-1 mapping between your model as well as GraphQL types. Here's an example GraphQL Type:
type User Type User's ID! "! "!" is the initial name which must be used. Stringlastname Email:: string username: String todos: [Todo] # Todo is a completely different GraphQL kind
Queries
GraphQL Query defines all the query queries clients can use the GraphQL API. Clients should build the root querythat includes all of the currently running queries using the protocol.
Below, we'll identify and match the query with the RESTful API which is compatible with them:
Type RootQuery user(id ID) User # corresponds to GET API/users/:idUsers: # corresponds to the API/users todostodo(id: ID! ): Todo # Corresponds to GET /api/todos/:id todos: [Todo] # Corresponds to GET /api/todos
Mutations
If GraphQL is one of the GET request, then the mutations include POST, the PUT, the PATCH, and the DELETE request that modify GraphQL API.
We'll combine all changes into one RootMutation to show:
type RootMutation *
You may have noticed the use of the -input types to define the types of transformations like the input of the user, TodoInput. It is always a good idea to define input types before designing or modifying your source.
You can define Input varieties like these:
Input UserInput: firstname String! Lastname: String Email String! username: String!
Resolvers
Create a new resolvers.js file and include the following code into it:
import sequelize from '../models'; export default function resolvers () const models = sequelize.models; return // Resolvers for Queries RootQuery: user (root, id , context) return models.User.findById(id, context); , users (root, args, context) return models.User.findAll(, context); , User: todos (user) return user.getTodos(); , // Resolvers for Mutations RootMutation: createUser (root, input , context) return models.User.create(input, context); , updateUser (root, id, input , context) return models.User.update(input, ...context, where: id ); , removeUser (root, id , context) return models.User.destroy(input, ...context, where: id ); , // ... Resolvers for Todos go here ; }
Schema
GraphQL schema determines the information GraphQL exposes to the world. Thus, the kinds, queries, and mutations will be in the schema and made available to the public.
Below are the steps to reveal kinds, queries and changes to the public:
schema query: RootQuery mutation: RootMutation
The script that we have created above have added to it the RootQuery as well as RootMutation which were developed previously in order to make them open to all the world.
What does GraphQL Work with Nodejs and Expressjs
GraphQL offers an implementation of the majority of programming languages, and Node.js is not an exception. The official GraphQL site contains an article that covers JavaScript support and there are other variants of GraphQL which make the writing and programming process in GraphQL easy.
GraphQL Apollo provides an implementation for Node.js and Express.js and allows users to begin using GraphQL.
It will be taught how to design and develop your first GraphQL application using Nodes.js as well as the Express.js backend framework with GraphQL Apollo in the following part.
Setting up GraphQL With the help of Express.js
Constructing a GraphQL API server using Express.js is an easy way for newbies to get started. In this article, we'll go over the steps to create an GraphQL server.
Create a New Project With Express
Set up a folder in your project, and then install Express.js by using the following command:
cd && npm init -y npm installation express
This command creates the brand new package.json file and it will also install the Express.js library within your application.
Do you want to learn the ways we have increased the number of visitors we have by 1,000?
Join over 20,000 people to get our email each week, which is packed with insider WordPress information!
Next, we will organize our work in the way depicted in the following image. The application will comprise a number of modules used in order to perform the duties of the program, such as user, task, etc.
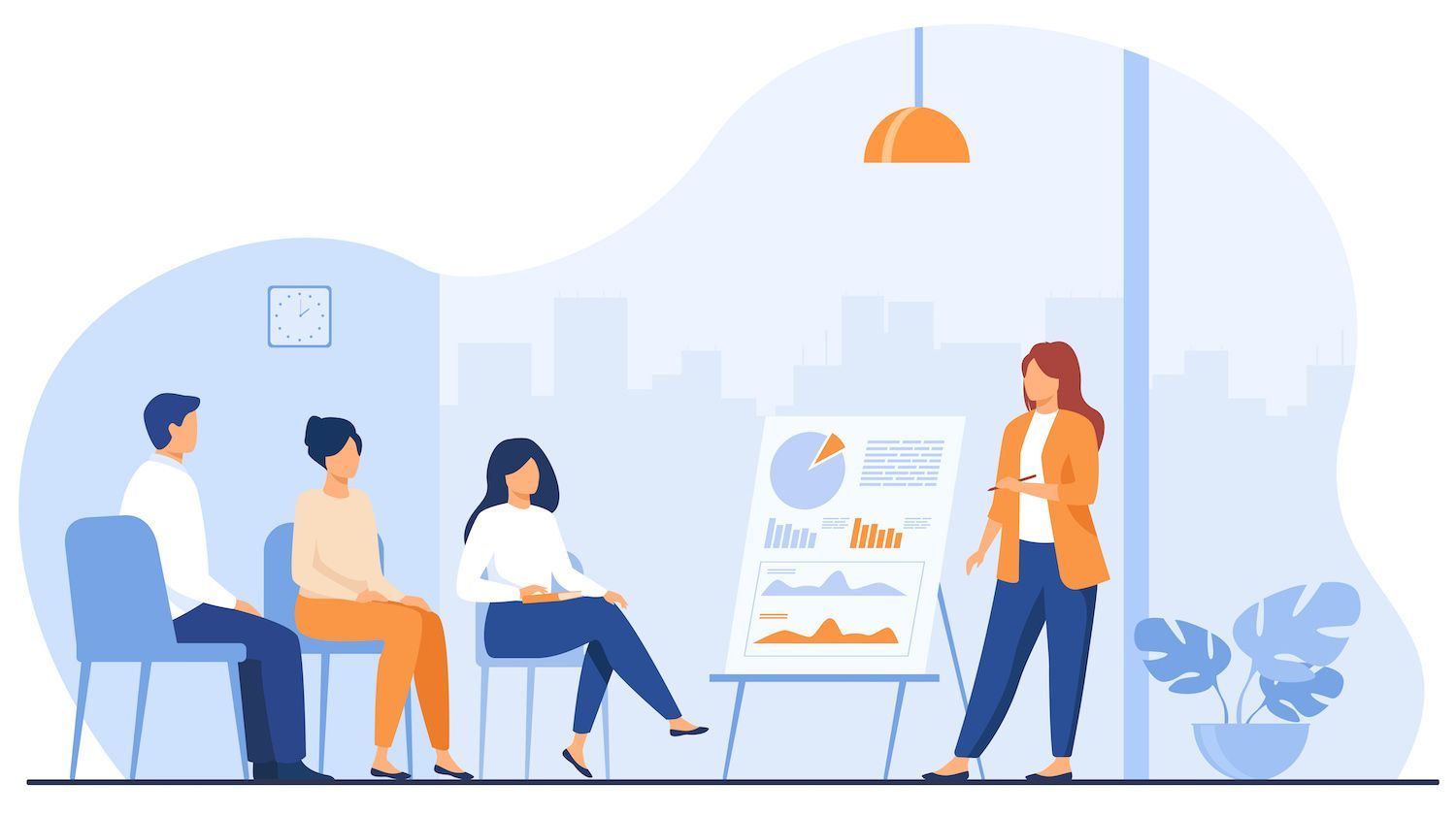
Initialize GraphQL
Set up the GraphQL Express.js dependencies. The following command is needed for installation:
npm install apollo-server-express graphql @graphql-tools/schema --save
The method of making Schemas and Types
Now, we will create index.jsfile. index.jsfile inside the module folder. After that, we'll add the code snippet below:
const gql = require('apollo-server-express'); const users = require('./users'); const todos = require('./todos'); const GraphQLScalarType = require('graphql'); const makeExecutableSchema = require('@graphql-tools/schema'); const typeDefs = gql` scalar Time type Query getVersion: String! type Mutation version: String! `; const timeScalar = new GraphQLScalarType( name: 'Time', description: 'Time custom scalar type', serialize: (value) => value, ); const resolvers = Time: timeScalar, Query: getVersion: () => `v1`, , ; const schema = makeExecutableSchema( typeDefs: [typeDefs, users.typeDefs, todos.typeDefs], resolvers: [resolvers, users.resolvers, todos.resolvers], ); module.exports = schema;
Code Walkthrough
Let's look at this code-snippet, and break it down
Step 1.
We then download the required libraries and set up the standard types of query and mutation. Both query and mutation are an instance of GraphQL API at present. However, we will extend the query as well as the mutation to incorporate more schemas in the future.
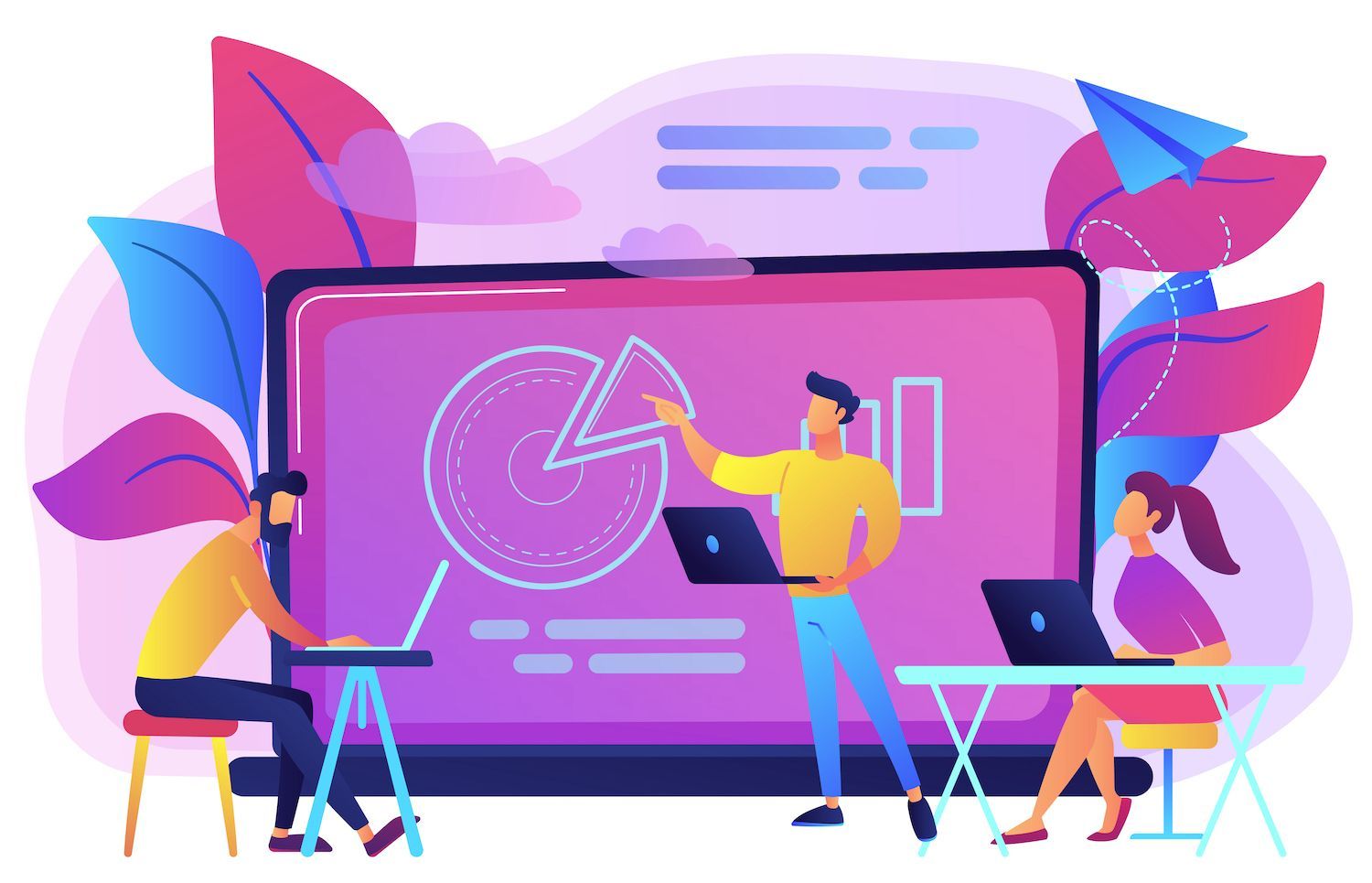
Step 2.
Then, we created a new type of scalar that represents time, and our first resolver for the query and the transformation made earlier. Additionally, we generated a schema using this createExecutableEchema function.
The schema generated includes the schemas that we have imported, and will add additional schemas in the future as we create and will then take them into the system.
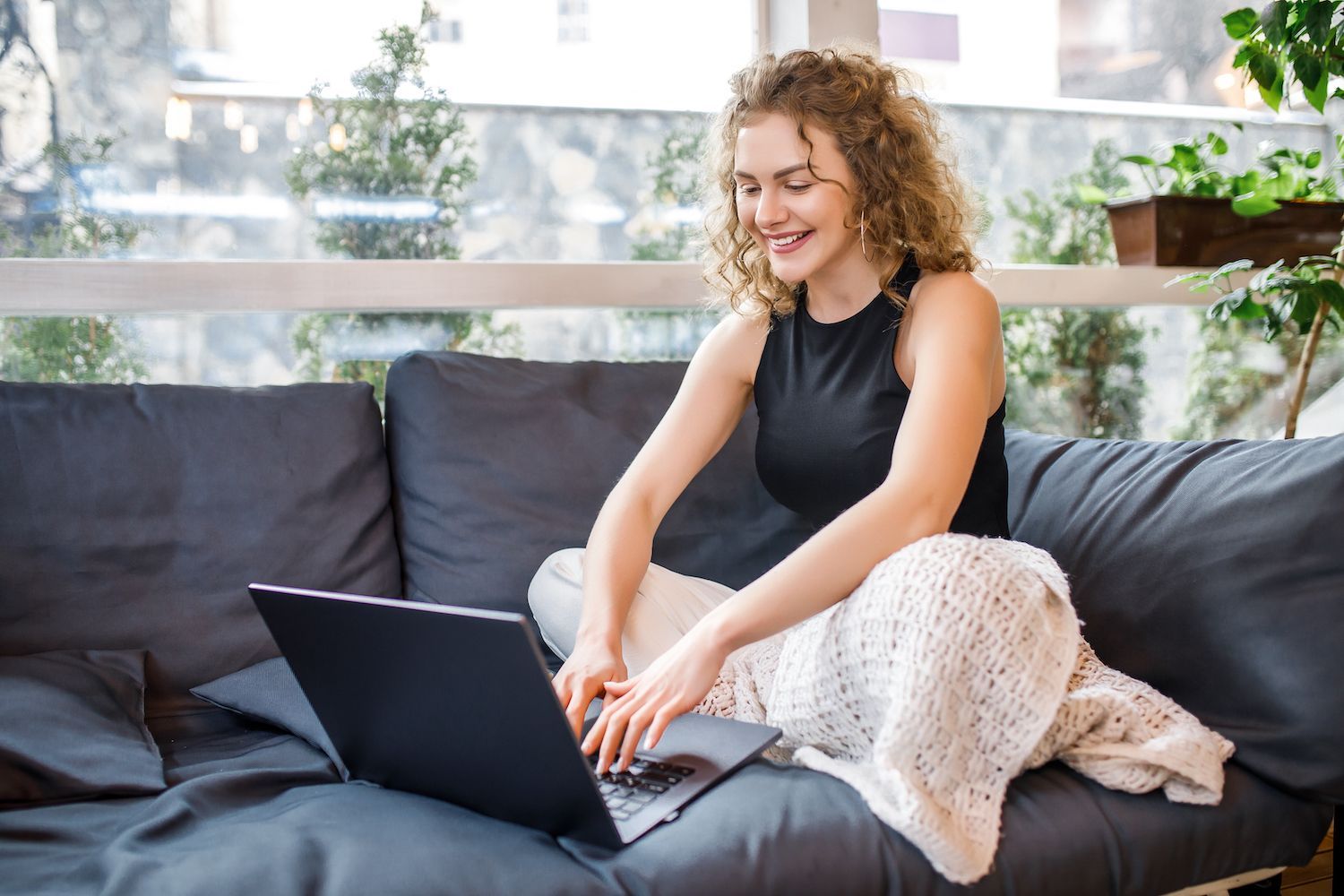
This example code shows how we have imported various schemas into the makeExecutableEchema method. This method helps in creating an application that is organized to deal with complexity. The next step is to create our own schemas built on the Todo as well as User schemas we brought in.
Creating Todo Schema
The Todo schema outlines the fundamental operations that users of the application can perform. The schema below executes the Todo operations CRUD.
const gql = require('apollo-server-express'); const createTodo = require('./mutations/create-todo'); const updateTodo = require('./mutations/update-todo'); const removeTodo = require('./mutations/delete-todo'); const todo = require('./queries/todo'); const todos = require('./queries/todos'); const typeDefs = gql` type Todo id: ID! title: String description: String user: User input CreateTodoInput title: String! description: String isCompleted: Boolean input UpdateTodoInput title: String description: String isCompleted: Boolean *do; // Implement resolvers for the schema fieldsconst resolvers = Resolvers to resolve queriesQuery such as todo, todos and// Resolvers for Mutations Mutation: createTodo, updateTodo, removeTodo, , ; Module.exports = typeDefs;module.exports = typeDefs, resolvers ;
Code Walkthrough
Let's work through this code-snippet, and break it down:
Step 1.
Then, we developed a structure to manage our tasks through the use of GraphQL forms, input as well as expanding. Extension keyword extensions keyword allows you to create additional queries or mutations in the current base query as well as the mutation that the above-mentioned transformation.
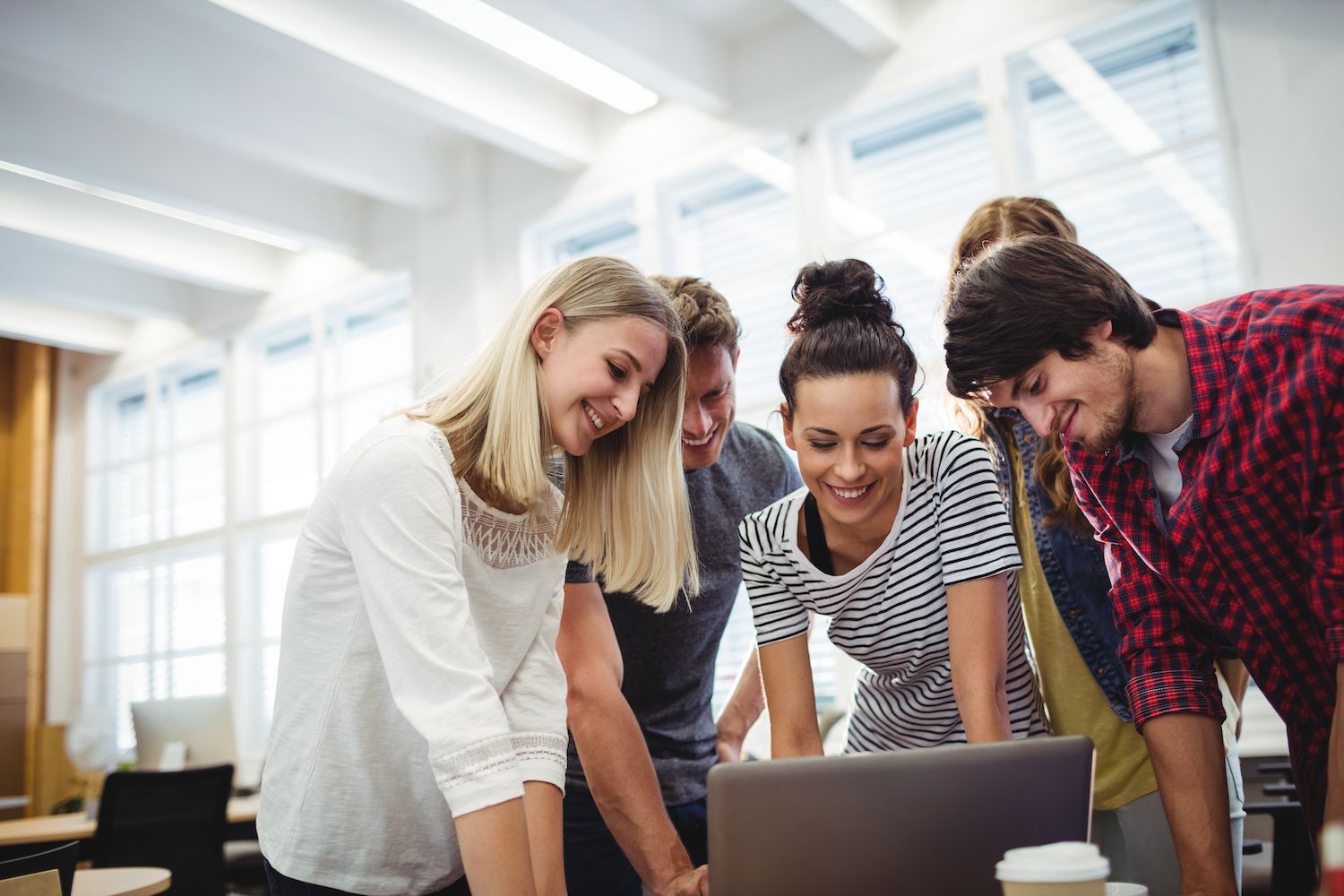
Step 2:
This is the resolver we developed, that can be used to find the right information whenever a particular demand or modification is pointed to as.
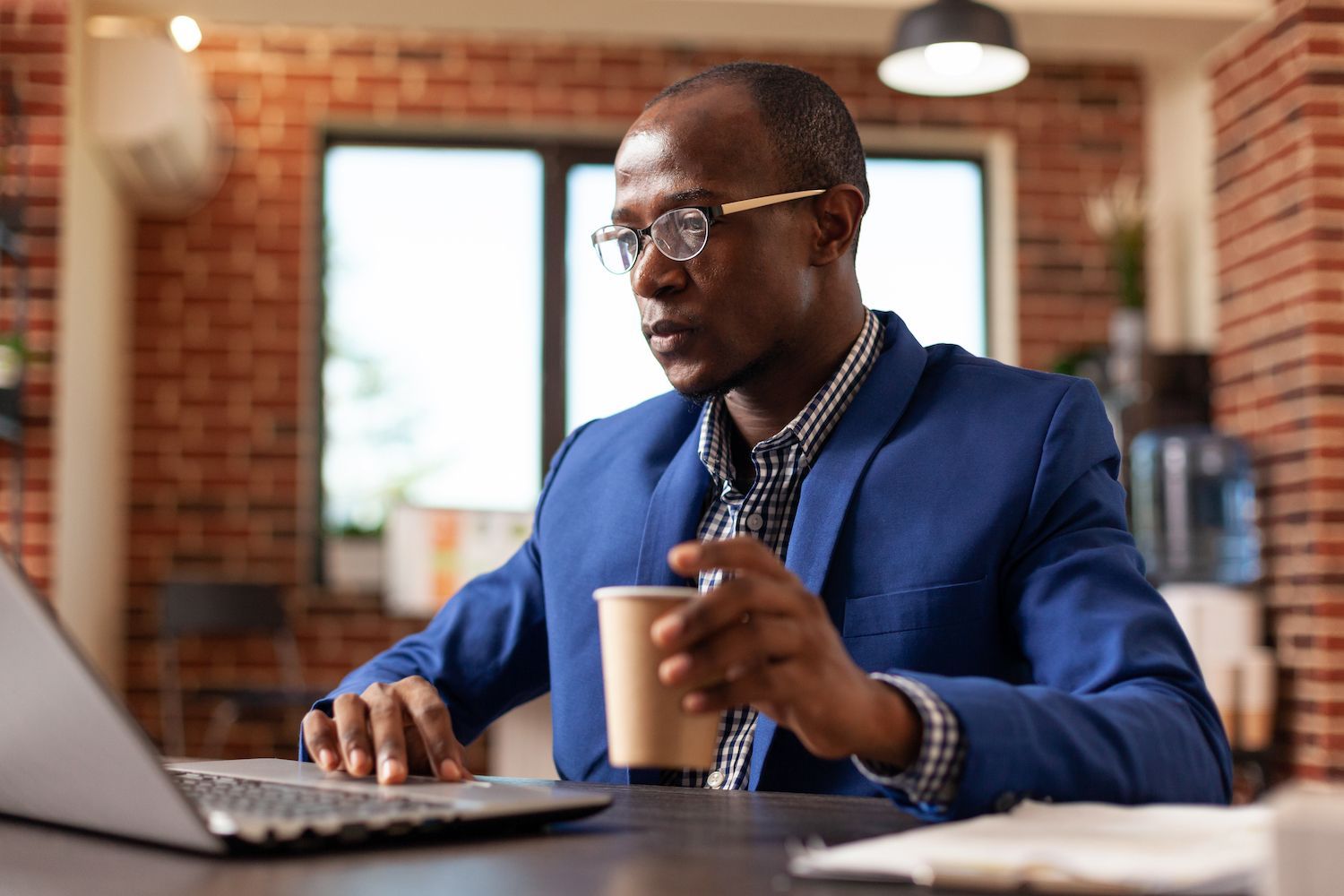
When we install the resolver function it is possible to create individual strategies to manage managing the database as well as the business logic as in create-todo.js example.
Create a create-user.js file in the ./mutations
folder and integrate the business logic to create the brand-new Todo in your database.
const models = require('../../../models'); module.exports = async (root, input , context) => return models.todos.push( ...input ); ;
The above code is a simplified way of creating the new Todo within our database by using the ORM Sequelize. It is possible to learn more on Sequelize as well as how to create it with Node.js.
You can follow this same procedure to build different schemas that are based on the requirements of your application or you can transfer the entire application to GitHub.
The next step is to make a server using Express.js and launch the new Todo application by using GraphQL along with Node.js
Configure and start run the Server
Lastly, we will set up our server using the apollo-server-express library we install earlier and configure it.
The apollo-server-express is a simple wrapper of Apollo Server for Express.js, It's recommended because it has been developed to fit in Express.js development.
Using the examples we discussed above, let's configure the Express.js server to work with the newly installed apollo-server-express.
Create server.js file in your root directory. Copy the server.js file in the root directory. Copy this code to it:
const express = require('express'); const ApolloServer = require('apollo-server-express'); const schema = require('./modules'); const app = express(); async function startServer() const server = new ApolloServer( schema ); await server.start(); server.applyMiddleware( app ); startServer(); app.listen( port: 3000 , () => console.log(`Server ready at http://localhost:3000`) );
The code you have seen above indicates that you've made it through the process of creating your very initial CRUD GraphQL server for Todos as well as Users. You can start your development server and access the playground using http://localhost:3000/graphql. If everything goes as planned, you should be presented with the screen following:
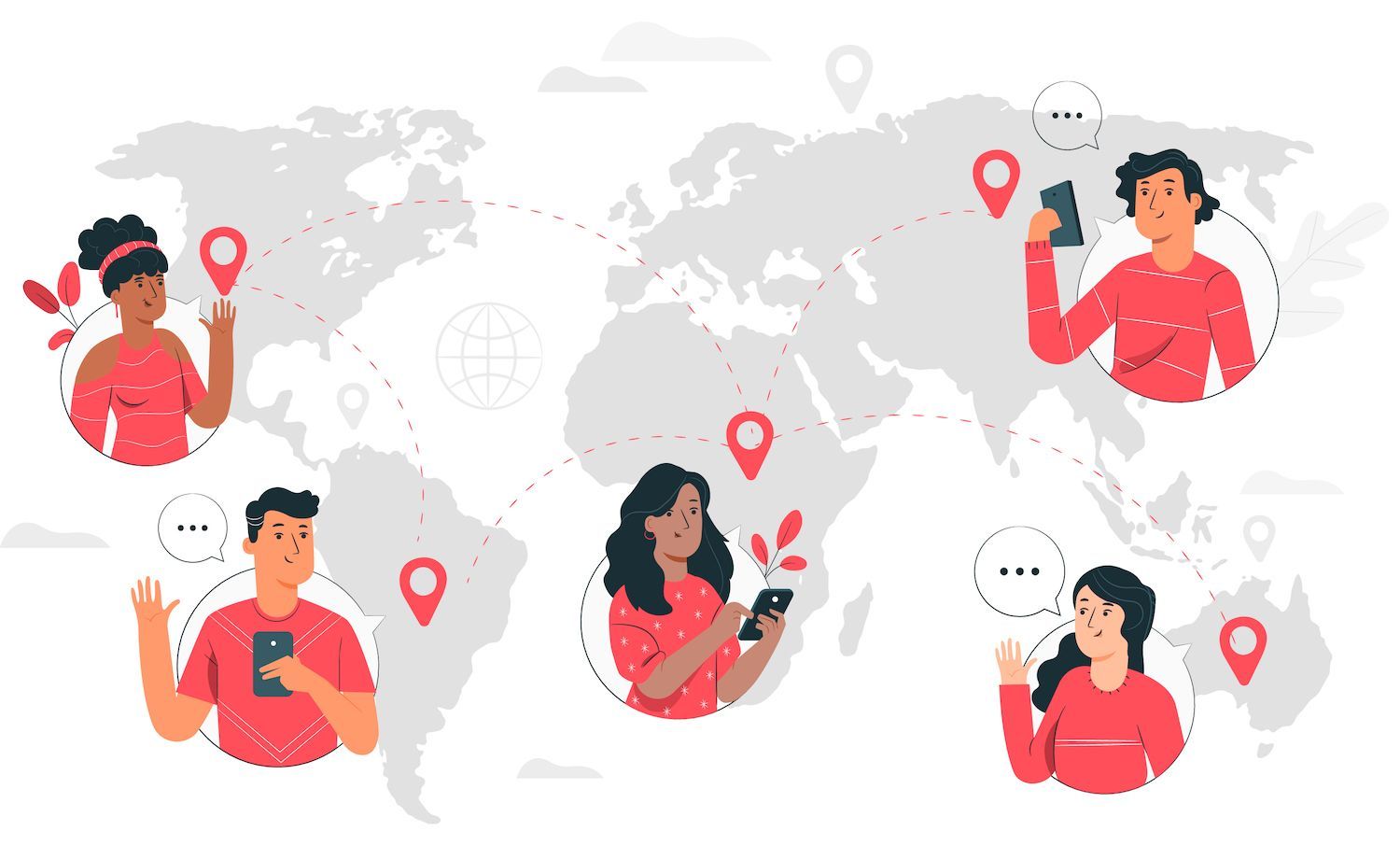
Summary
GraphQL is modern technology used by Facebook that makes easier the task of creating vast-scale APIs that use RESTful architectural designs.
This article has provided an overview of GraphQL and showed how you can build your very own GraphQL API with Express.js.
Let us know the things you have built by using GraphQL.
- Easy to set up and manage My dashboard. My dashboard
- Support is available 24/7.
- The top Google Cloud Platform hardware and network driven by Kubernetes for maximum scalability
- High-end Cloudflare integration that improves speed as well as security
- Reach to the world's audience with more than 35 data centers and more than 275 POPs across all over the globe.
This post was first seen on here